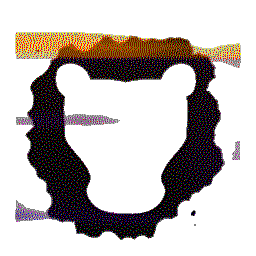 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include "core/audit/AuditEntry_odb.h"
25 #include "core/auth/AccessPoint_odb.h"
26 #include "core/auth/Door_odb.h"
27 #include "core/auth/Group_odb.h"
28 #include "core/auth/User_odb.h"
29 #include "core/auth/Zone_odb.h"
30 #include "core/credentials/Credential_odb.h"
33 #include "tools/Schedule_odb.h"
35 #include <odb/database.hxx>
55 auto tracer = assert_cast<db::DatabaseTracer *>(
database_->tracer());
56 return tracer->count();
66 if (!grp && flags & Flag::THROW_IF_NOT_FOUND)
76 if (!user && flags & Flag::THROW_IF_NOT_FOUND)
87 if (!ugm && flags & Flag::THROW_IF_NOT_FOUND)
98 if (!cred && flags & Flag::THROW_IF_NOT_FOUND)
109 if (!sched && flags & Flag::THROW_IF_NOT_FOUND)
120 if (!door && flags & Flag::THROW_IF_NOT_FOUND)
131 if (!zone && flags & Flag::THROW_IF_NOT_FOUND)
143 if (!audit && flags & Flag::THROW_IF_NOT_FOUND)
157 if (!ap && flags & Flag::THROW_IF_NOT_FOUND)
168 if (!ap && flags & Flag::THROW_IF_NOT_FOUND)
173 template <
typename T>
174 static void persist_impl(
const DBPtr db, T &&obj)
177 ASSERT_LOG(obj.id() == 0,
"Object is probably already persisted.");
178 db->persist(std::forward<T>(obj));
182 template <
typename T>
183 static void update_impl(
const DBPtr db, T &&obj)
186 db->update(std::forward<T>(obj));
192 persist_impl(
database_, assert_cast<Audit::AuditEntry &>(ientry));
197 update_impl(
database_, assert_cast<Audit::AuditEntry &>(ientry));
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
update::IUpdatePtr find_update_by_id(const update::UpdateId &id, Flag f=Flag::DEFAULT)
#define ASSERT_LOG(cond, msg)
Auth::IDoorPtr find_door_by_id(const Auth::DoorId &id, Flag f=Flag::DEFAULT)
size_t operation_count() const
Return the number of operation against the database.
std::shared_ptr< User > UserPtr
std::shared_ptr< IUpdate > IUpdatePtr
unsigned long AuditEntryId
std::shared_ptr< odb::database > DBPtr
std::shared_ptr< IAuditEntry > IAuditEntryPtr
std::shared_ptr< IAccessPoint > IAccessPointPtr
void update(Audit::IAuditEntry &)
Update the object.
Audit::IAuditEntryPtr find_audit_by_id(const Audit::AuditEntryId &id, Flag f=Flag::DEFAULT)
Tools::ISchedulePtr find_schedule_by_id(const Tools::ScheduleId &id, Flag f=Flag::DEFAULT)
A Zone is a container for doors and other zone.
This is the header file for a generated source file, GitSHA1.cpp.
Cred::ICredentialPtr find_credential_by_id(const Cred::CredentialId &id, Flag f=Flag::DEFAULT)
unsigned long UserGroupMembershipId
std::shared_ptr< IDoor > IDoorPtr
std::shared_ptr< Group > GroupPtr
A authentication group regroup users that share permissions.
An ODB enabled credential object.
Auth::UserGroupMembershipPtr find_membership_by_id(const Auth::UserGroupMembershipId &id, Flag f=Flag::DEFAULT)
std::shared_ptr< UserGroupMembership > UserGroupMembershipPtr
Auth::GroupPtr find_group_by_id(const Auth::GroupId &id, Flag f=Flag::DEFAULT)
Retrieve a group by its id.
Auth::IAccessPointPtr find_access_point_by_id(const Auth::AccessPointId &id, Flag f=Flag::DEFAULT)
Base interface to Audit object.
std::shared_ptr< ICredential > ICredentialPtr
unsigned long CredentialId
DBPtr db() const
Simply returns the underlying database pointer.
Auth::UserPtr find_user_by_id(const Auth::UserId &id, Flag f=Flag::DEFAULT)
Find a user by its id.
std::shared_ptr< IZone > IZonePtr
void persist(Audit::IAuditEntry &)
Persist an audit entry object.
Implementation of IAuditEntry, backed by ODB.
Auth::IZonePtr find_zone_by_id(const Auth::ZoneId &id, Flag f=Flag::DEFAULT)
unsigned long AccessPointId
Describe the membership of an User with regroup to a Group.