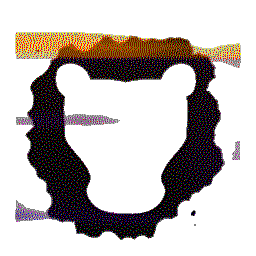 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <boost/date_time/posix_time/posix_time.hpp>
167 virtual boost::posix_time::ptime
timestamp()
const = 0;
183 virtual size_t version()
const = 0;
virtual size_t version() const =0
Returns the ODB version of the object.
virtual void author(Auth::UserPtr user)=0
Set the author of the entry.
std::shared_ptr< User > UserPtr
virtual void set_parent(IAuditEntryPtr parent)=0
Set parent as the parent audit entry for this entry.
unsigned long AuditEntryId
std::shared_ptr< IAuditEntry > IAuditEntryPtr
virtual void finalize()=0
Mark the entry as finalized, and update it wrt the database.
virtual size_t children_count() const =0
Returns the number of children that this entry has.
virtual void remove_parent()=0
Remove the parent-child relationship between this entry and its parent.
This is the header file for a generated source file, GitSHA1.cpp.
virtual const EventMask & event_mask() const =0
Retrieve the current event mask.
virtual boost::posix_time::ptime timestamp() const =0
Retrieve unix timestamp.
virtual std::string generate_description() const
Generate a description for this event.
FlagSet< EventType > EventMask
virtual IAuditEntryPtr parent() const =0
Retrieve the parent of this entry.
virtual Auth::UserId author_id() const =0
Retrieve the user id of the author of this entry.
virtual void reload()=0
Reload the object from the database.
Base interface to Audit object.
virtual AuditEntryId id() const =0
Retrieve the identifier of the entry.
virtual bool finalized() const =0
Is this entry finalized.