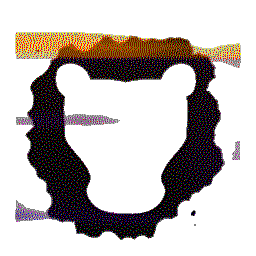 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
40 #pragma db object optimistic
44 Schedule(
const std::string &sched_name =
"");
48 const std::string &
name()
const override;
51 is_in_schedule(
const std::chrono::system_clock::time_point &tp)
const override;
57 void name(
const std::string &)
override;
65 std::vector<SingleTimeFrame>
timeframes()
const override;
71 std::vector<ScheduleMappingPtr>
mapping()
const override;
77 friend class ::Leosac::TestAccess;
82 #pragma db id_column("schedule_id") value_column("timeframe")
90 #pragma db id_column("schedule_id") value_column("schedule_mapping_id")
91 std::vector<Tools::ScheduleMappingPtr>
mapping_;
This is the header file for a generated source file, GitSHA1.cpp.