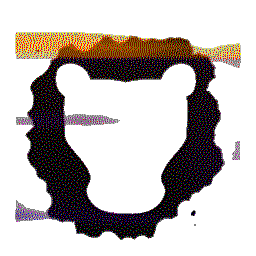 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <odb/callback.hxx>
43 static void validate(
const Zone &z, std::set<ZoneId> &zone_ids);
59 #pragma db object callback(validation_callback) optimistic
66 virtual std::string
alias()
const override;
70 virtual void alias(
const std::string &
alias)
override;
72 virtual void description(
const std::string &desc)
override;
78 virtual std::vector<ZoneLPtr>
children()
const override;
80 virtual std::vector<DoorLPtr>
doors()
const override;
107 #pragma db value_not_null
110 #pragma db value_not_null
113 #pragma db value_not_null inverse(children_)
virtual Type type() const override
virtual void add_door(DoorLPtr door) override
virtual std::vector< ZoneLPtr > children() const override
Retrieve the children zones.
virtual std::string alias() const override
virtual void clear_doors() override
virtual std::vector< DoorLPtr > doors() const override
Retrieve the doors associated with the zones.
std::vector< ZoneLPtr > children_
std::vector< ZoneLWPtr > parents_
virtual std::string description() const override
A Zone is a container for doors and other zone.
This is the header file for a generated source file, GitSHA1.cpp.
static void validate_type(IZone::Type value)
Validate that the integer value of the type is a correct type for a zone.
virtual void clear_children() override
virtual ZoneId id() const override
virtual void add_child(ZoneLPtr zone) override
void validation_callback(odb::callback_event e, odb::database &) const
Callback function called by ODB before/after database operation against a Zone object.
This is class that can be used to access some object's internal.
odb::lazy_shared_ptr< Zone > ZoneLPtr
static void validate(const Zone &z, std::set< ZoneId > &zone_ids)
Perform validation of the zone.
odb::lazy_shared_ptr< Door > DoorLPtr
std::vector< DoorLPtr > doors_