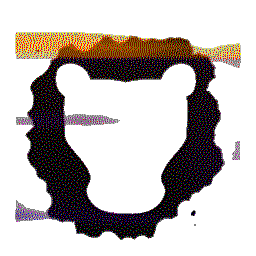 |
Leosac
0.8.0
Open Source Access Control
|
#include <cassert>
#include <csignal>
#include <iostream>
#include <spdlog/spdlog.h>
#include <sstream>
#include <string>
Go to the source code of this file.
|
#define | FUNCTION_NAME_MACRO __FUNCTION__ |
|
#define | BUILD_STR(param) |
| Internal macro. More...
|
|
#define | DEBUG_0(msg) |
| See "Internal macros documentation". More...
|
|
#define | DEBUG_1(msg, loggers) |
| See "Internal macros documentation". More...
|
|
#define | DEBUG_X(trash, msg, loggers, targetMacro, ...) targetMacro |
| See "Internal macros documentation". More...
|
|
#define | DEBUG(...) |
| Debug macro. More...
|
|
#define | INFO_0(msg) |
| See "Internal macros documentation". More...
|
|
#define | INFO_1(msg, loggers) |
| See "Internal macros documentation". More...
|
|
#define | INFO_X(trash, msg, loggers, targetMacro, ...) targetMacro |
| See "Internal macros documentation". More...
|
|
#define | INFO(...) |
| Information macro. More...
|
|
#define | WARN_0(msg) |
| See "Internal macros documentation". More...
|
|
#define | WARN_1(msg, loggers) |
| See "Internal macros documentation". More...
|
|
#define | WARN_X(trash, msg, loggers, targetMacro, ...) targetMacro |
| See "Internal macros documentation". More...
|
|
#define | WARN(...) |
| Warning macro. More...
|
|
#define | ERROR_0(msg) |
| See "Internal macros documentation". More...
|
|
#define | ERROR_1(msg, loggers) |
| See "Internal macros documentation". More...
|
|
#define | ERROR_X(trash, msg, loggers, targetMacro, ...) targetMacro |
| See "Internal macros documentation". More...
|
|
#define | ERROR(...) |
| Error macro. More...
|
|
#define | ASSERT_LOG(cond, msg) |
|
|
enum | LogLevel {
CRIT = spdlog::level::critical,
ERROR = spdlog::level::err,
WARN = spdlog::level::warn,
INFO = spdlog::level::info,
DEBUG = spdlog::level::debug
} |
|
◆ ASSERT_LOG
#define ASSERT_LOG |
( |
|
cond, |
|
|
|
msg |
|
) |
| |
Value: do \
{ \
if (!(cond)) \
{ \
ERROR(msg); \
ERROR("Assertion failed in " << __FILE__ << " --> " \
<< ". Aborting."); \
raise(SIGABRT); \
} \
} while (false)
Definition at line 190 of file log.hpp.
◆ BUILD_STR
#define BUILD_STR |
( |
|
param | ) |
|
Value: [&](void) { \
std::stringstream logger_macro_ss__; \
logger_macro_ss__ << param; \
return logger_macro_ss__.str(); \
}()
Internal macro.
It is used to create an anonymous lambda to allow the logging macros parameter to behave like a stringstream.
Definition at line 63 of file log.hpp.
◆ DEBUG
Value:
DEBUG_NO_PARAM(__VA_ARGS__), )
Debug macro.
Issue a log message with DEBUG level
Definition at line 94 of file log.hpp.
◆ DEBUG_0
Value:
See "Internal macros documentation".
Definition at line 74 of file log.hpp.
◆ DEBUG_1
#define DEBUG_1 |
( |
|
msg, |
|
|
|
loggers |
|
) |
| |
Value:
See "Internal macros documentation".
Definition at line 81 of file log.hpp.
◆ DEBUG_X
#define DEBUG_X |
( |
|
trash, |
|
|
|
msg, |
|
|
|
loggers, |
|
|
|
targetMacro, |
|
|
|
... |
|
) |
| targetMacro |
See "Internal macros documentation".
Definition at line 88 of file log.hpp.
◆ ERROR
Value:
ERROR_NO_PARAM(__VA_ARGS__), )
Error macro.
Issue a log message with ERROR level
Definition at line 178 of file log.hpp.
◆ ERROR_0
Value:
See "Internal macros documentation".
Definition at line 158 of file log.hpp.
◆ ERROR_1
#define ERROR_1 |
( |
|
msg, |
|
|
|
loggers |
|
) |
| |
Value:
See "Internal macros documentation".
Definition at line 165 of file log.hpp.
◆ ERROR_X
#define ERROR_X |
( |
|
trash, |
|
|
|
msg, |
|
|
|
loggers, |
|
|
|
targetMacro, |
|
|
|
... |
|
) |
| targetMacro |
See "Internal macros documentation".
Definition at line 172 of file log.hpp.
◆ FUNCTION_NAME_MACRO
#define FUNCTION_NAME_MACRO __FUNCTION__ |
Macro used to get the name of the function that called the logging macro. GNU compilers provide a nice macro the get a more detailed name of a function. Otherwise we fallback to a more widespread macro.
Definition at line 55 of file log.hpp.
◆ INFO
Value:
INFO_NO_PARAM(__VA_ARGS__), )
Information macro.
Issue a log message with INFO level.
Definition at line 122 of file log.hpp.
◆ INFO_0
Value:
See "Internal macros documentation".
Definition at line 102 of file log.hpp.
◆ INFO_1
#define INFO_1 |
( |
|
msg, |
|
|
|
loggers |
|
) |
| |
Value:
See "Internal macros documentation".
Definition at line 109 of file log.hpp.
◆ INFO_X
#define INFO_X |
( |
|
trash, |
|
|
|
msg, |
|
|
|
loggers, |
|
|
|
targetMacro, |
|
|
|
... |
|
) |
| targetMacro |
See "Internal macros documentation".
Definition at line 116 of file log.hpp.
◆ WARN
Value:
WARN_NO_PARAM(__VA_ARGS__), )
Warning macro.
Issue a log message with WARN level.
Definition at line 150 of file log.hpp.
◆ WARN_0
Value:
See "Internal macros documentation".
Definition at line 130 of file log.hpp.
◆ WARN_1
#define WARN_1 |
( |
|
msg, |
|
|
|
loggers |
|
) |
| |
Value:
See "Internal macros documentation".
Definition at line 137 of file log.hpp.
◆ WARN_X
#define WARN_X |
( |
|
trash, |
|
|
|
msg, |
|
|
|
loggers, |
|
|
|
targetMacro, |
|
|
|
... |
|
) |
| targetMacro |
See "Internal macros documentation".
Definition at line 144 of file log.hpp.
◆ LogLevel
Enumerator |
---|
CRIT | |
ERROR | |
WARN | |
INFO | |
DEBUG | |
Definition at line 29 of file log.hpp.
#define INFO(...)
Information macro.
#define BUILD_STR(param)
Internal macro.
#define ERROR_0(msg)
See "Internal macros documentation".
#define WARN_X(trash, msg, loggers, targetMacro,...)
See "Internal macros documentation".
#define DEBUG_1(msg, loggers)
See "Internal macros documentation".
#define FUNCTION_NAME_MACRO
#define DEBUG_X(trash, msg, loggers, targetMacro,...)
See "Internal macros documentation".
#define INFO_1(msg, loggers)
See "Internal macros documentation".
#define ERROR_1(msg, loggers)
See "Internal macros documentation".
#define ERROR(...)
Error macro.
#define WARN_1(msg, loggers)
See "Internal macros documentation".
#define WARN(...)
Warning macro.
#define ERROR_X(trash, msg, loggers, targetMacro,...)
See "Internal macros documentation".
#define DEBUG_0(msg)
See "Internal macros documentation".
#define INFO_X(trash, msg, loggers, targetMacro,...)
See "Internal macros documentation".
#define DEBUG(...)
Debug macro.
#define INFO_0(msg)
See "Internal macros documentation".
void log(const std::string &log_msg, int, const char *, const char *, LogLevel level)
#define WARN_0(msg)
See "Internal macros documentation".