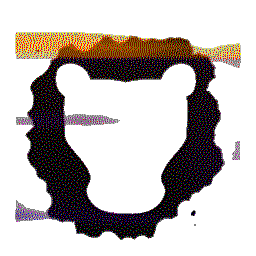 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
68 Flag f = Flag::DEFAULT);
71 Flag f = Flag::DEFAULT);
74 Flag f = Flag::DEFAULT);
81 Flag f = Flag::DEFAULT);
84 Flag f = Flag::DEFAULT);
87 Flag f = Flag::DEFAULT);
update::IUpdatePtr find_update_by_id(const update::UpdateId &id, Flag f=Flag::DEFAULT)
Auth::IDoorPtr find_door_by_id(const Auth::DoorId &id, Flag f=Flag::DEFAULT)
size_t operation_count() const
Return the number of operation against the database.
std::shared_ptr< User > UserPtr
std::shared_ptr< IUpdate > IUpdatePtr
unsigned long AuditEntryId
std::shared_ptr< odb::database > DBPtr
std::shared_ptr< IAuditEntry > IAuditEntryPtr
std::shared_ptr< IAccessPoint > IAccessPointPtr
Provides various database-related services to consumer.
void update(Audit::IAuditEntry &)
Update the object.
Audit::IAuditEntryPtr find_audit_by_id(const Audit::AuditEntryId &id, Flag f=Flag::DEFAULT)
Tools::ISchedulePtr find_schedule_by_id(const Tools::ScheduleId &id, Flag f=Flag::DEFAULT)
This is the header file for a generated source file, GitSHA1.cpp.
Cred::ICredentialPtr find_credential_by_id(const Cred::CredentialId &id, Flag f=Flag::DEFAULT)
unsigned long UserGroupMembershipId
std::shared_ptr< IDoor > IDoorPtr
std::shared_ptr< Group > GroupPtr
Auth::UserGroupMembershipPtr find_membership_by_id(const Auth::UserGroupMembershipId &id, Flag f=Flag::DEFAULT)
std::shared_ptr< UserGroupMembership > UserGroupMembershipPtr
Auth::GroupPtr find_group_by_id(const Auth::GroupId &id, Flag f=Flag::DEFAULT)
Retrieve a group by its id.
Auth::IAccessPointPtr find_access_point_by_id(const Auth::AccessPointId &id, Flag f=Flag::DEFAULT)
Base interface to Audit object.
std::shared_ptr< ICredential > ICredentialPtr
unsigned long CredentialId
DBPtr db() const
Simply returns the underlying database pointer.
Auth::UserPtr find_user_by_id(const Auth::UserId &id, Flag f=Flag::DEFAULT)
Find a user by its id.
std::shared_ptr< IZone > IZonePtr
void persist(Audit::IAuditEntry &)
Persist an audit entry object.
Auth::IZonePtr find_zone_by_id(const Auth::ZoneId &id, Flag f=Flag::DEFAULT)
unsigned long AccessPointId