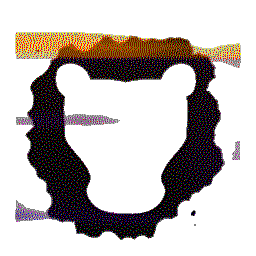 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
29 #pragma db object optimistic polymorphic
31 public std::enable_shared_from_this<AccessPoint>
37 const std::string &
alias()
const override;
39 void alias(
const std::string &new_alias)
override;
67 #pragma db inverse(access_point_)
79 #pragma db value_not_null
DoorId door_id() const override
std::shared_ptr< AccessPointUpdate > AccessPointUpdatePtr
std::string controller_module() const override
The name of the module that manages the access point.
This is the header file for a generated source file, GitSHA1.cpp.
std::weak_ptr< Door > door_
std::shared_ptr< IDoor > IDoorPtr
IDoorPtr door() const override
AccessPointId id() const override
This is class that can be used to access some object's internal.
const std::string & alias() const override
std::vector< AccessPointUpdateLPtr > updates_
The history of the updates performed against the access-point.
std::string controller_module_
Which module is responsible for this access point.
const std::string & description() const override
An interface for access point.
void attach_update(AccessPointUpdatePtr)
Attach a new update object to the access-point.
unsigned long AccessPointId