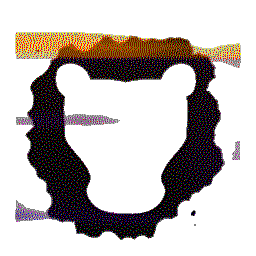 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
34 #pragma db object optimistic
41 virtual std::string
alias()
const override;
45 virtual void alias(
const std::string &
alias)
override;
47 virtual void description(
const std::string &desc)
override;
55 virtual std::vector<Tools::ScheduleMappingLWPtr>
lazy_mapping()
const override;
83 #pragma db value_not_null inverse(doors_)
std::shared_ptr< AccessPoint > access_point_
The access point that controls the door.
virtual std::string description() const override
void schedule_mapping_added(const Tools::ScheduleMappingPtr &sched_mapping)
A ScheduleMapping object has added this door as part of its mapping.
virtual AccessPointId access_point_id() const override
std::vector< Tools::ScheduleMappingLWPtr > schedules_mapping_
ScheduleMapping that maps this door.
std::shared_ptr< IAccessPoint > IAccessPointPtr
This is the header file for a generated source file, GitSHA1.cpp.
virtual std::vector< Tools::ScheduleMappingLWPtr > lazy_mapping() const override
Retrieve the lazy pointers to the ScheduleMapping objects that map this door.
virtual DoorId id() const override
virtual std::string alias() const override
This is class that can be used to access some object's internal.
virtual IAccessPointPtr access_point() const override
unsigned long AccessPointId