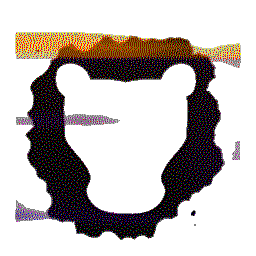 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
36 #pragma db object polymorphic optimistic
45 virtual std::string
alias()
const override;
55 virtual void alias(
const std::string &
id)
override;
59 virtual void description(
const std::string &str)
override;
65 virtual std::vector<Tools::ScheduleMappingLWPtr>
86 #pragma db value_not_null inverse(creds_)
94 friend class ::Leosac::TestAccess;
virtual Auth::UserLPtr owner() const override
Retrieve the owner of the credential.
virtual size_t odb_version() const override
Credentials are "optimistic" object (wrt ODB).
virtual CredentialId id() const override
Retrieve the identifier of the credential.
virtual std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const override
Retrieve the lazy_weak_ptr to ScheduleMapping that map this credential.
This is the header file for a generated source file, GitSHA1.cpp.
odb::lazy_shared_ptr< User > UserLPtr
Base interface for credential objects.
virtual const Auth::ValidityInfo & validity() const override
Retrieve validity status from the credential.
An ODB enabled credential object.
Auth::ValidityInfo validity_
void schedule_mapping_added(const Tools::ScheduleMappingPtr &sched_mapping)
The credential has been mapped by a schedule.
virtual std::string alias() const override
An alias for the credential.
unsigned long CredentialId
std::vector< Tools::ScheduleMappingLWPtr > schedules_mapping_
virtual Auth::UserId owner_id() const override
Returns the id of the owner, or 0 if there is no owner (or the owner has no id).
A simple class that stores (and can be queried for) the validity of some objects.
virtual std::string description() const override
An optional description / notes for the credential.