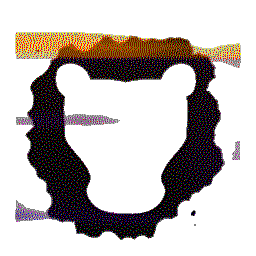 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
31 , config_manager_(k.config_manager())
32 , core_utils_(k.core_utils())
43 catch (
const std::exception &e)
49 std::cerr <<
"Unkown exception in ModuleManager destructor" << std::endl;
59 module_info.lib_->close();
82 assert(modinfo->
actor_ ==
nullptr);
83 assert(modinfo->
lib_);
84 using namespace Colorize;
88 char *(*module_name_fct)(void) =
89 (
char *(*)(void))modinfo->
lib_->getSymbol(
"get_module_name");
90 assert(module_name_fct);
91 std::string module_exported_name(module_name_fct());
93 if (modinfo->
name_ != module_exported_name)
95 std::stringstream error;
96 error <<
"Missconfiguration: Configured module name doesn't match the "
97 "name exported by the module. "
98 <<
"(" << modinfo->
name_ <<
" != " << module_exported_name <<
")";
103 void *symptr = modinfo->
lib_->getSymbol(
"start_module");
106 std::function<bool(zmqpp::socket *, boost::property_tree::ptree,
108 actor_fun = ((bool (*)(zmqpp::socket *, boost::property_tree::ptree,
111 auto new_module = std::unique_ptr<zmqpp::actor>(
112 new zmqpp::actor(std::bind(actor_fun, std::placeholders::_1,
115 modinfo->
actor_ = std::move(new_module);
118 <<
green(modinfo->
name_) <<
" initialized. (level = "
122 catch (std::exception &e)
125 <<
". See below for "
126 "exception information.");
129 "Unable to init module " +
red(modinfo->
name_) +
": " + e.what()));
142 WARN(
"Cannot find any module nammed " << name);
151 path_.push_back(dir);
157 std::string filename = cfg.get_child(
"file").data();
159 INFO(
"Attempting to load module nammed "
160 << module_name <<
" (shared lib file = " << filename <<
")");
161 for (
const std::string &path_entry :
path_)
164 if (UnixFs::fileExists(path_entry +
"/" + filename))
168 module_info.
name_ = module_name;
172 modules_.insert(std::move(module_info));
173 DEBUG(
"library file loaded (not init yet)");
177 ERROR(
"Could'nt load this module (file not found)");
181 std::shared_ptr<DynamicLibrary>
184 INFO(
"Loading library at: " << full_path);
185 std::shared_ptr<DynamicLibrary> lib(
new DynamicLibrary(full_path));
192 ERROR(
"FAILURE, full path was:{" << full_path <<
"}: " << e.
what());
218 INFO(
"Will now stop module " << modinfo->
name_ <<
" (Soft Stop: " << soft
223 modinfo->
actor_->stop(
false);
227 modinfo->
actor_->stop(
true);
228 modinfo->
actor_ =
nullptr;
235 INFO(
"Not stopping module " << modinfo->
name_
236 <<
" as it doesn't seem to run.");
250 WARN(
"Cannot find any module nammed " << name);
270 actor_ = std::move(o.actor_);
280 std::vector<std::string> ret;
285 ret.push_back(module.name_);
294 [&](
const ModuleInfo &m) { return m.name_ == name; });
313 int level_me = cfg_.load_config(name_).get<
int>(
"level", 100);
314 int level_o = cfg_.load_config(o.
name_).get<
int>(
"level", 100);
316 return level_me < level_o;
void print_exception(const std::exception &e, int level=0)
Recursively print the exception trace to std::cerr.
bool operator<(const ModuleInfo &o) const
virtual const char * what() const noexcept final
const boost::property_tree::ptree & load_config(const std::string &module) const
Return the stored configuration for a given module.
std::unique_ptr< zmqpp::actor > actor_
Actor object that runs the module code.
That class helps manage the configuration for the application and its module.
void log_exception(const std::exception &e, int level=0)
Recursively log exceptions using the logging macro.
ModuleManager(zmqpp::context &ctx, Leosac::Kernel &k)
Construct the module manager.
Wraps a dynamic library handler and provide methods to interact with it.
void initModules()
Actually call the init_module() function of each library we loaded.
Leosac::CoreUtilsPtr core_utils_
unix filesystem helper functions
This is the header file for a generated source file, GitSHA1.cpp.
void open(RelocationMode mode=RelocationMode::Lazy)
Attempts to open the shared library file so that we can access its symbols.
ModuleInfo * find_module_by_name(const std::string &name) const
std::vector< std::string > modules_names() const
Returns the list of the name of the loaded modules.
const std::vector< std::string > & get_module_path() const
Return the list of paths where we search for module.
std::string green(const T &in)
std::shared_ptr< DynamicLibrary > load_library_file(const std::string &full_path)
This will load (actually calling dlopen()) the library file located at full_path.
ModuleInfo(const Leosac::ConfigManager &cfg)
bool initModule(const std::string &name)
Attempt to find a module using its name, then load it.
Leosac::ConfigManager & config_manager_
std::set< ModuleInfo > modules_
std::string red(const T &in)
Internal helper struct that store informations related to module that are useful to the module manage...
void stopModules(bool soft=false)
Opposite of init module.
void addToPath(const std::string &dir)
Add a directory to a path.
bool has_module(const std::string &name) const
Do we have some informations about the module "name".
bool loadModule(const std::string &module_name)
Search the path and load a module based on a property tree for this module.
std::shared_ptr< CoreUtils > CoreUtilsPtr
bool stopModule(const std::string &name)
Stop a module by name and remove its config info from the config manager.
std::string name_
Name of the module, as specified in the configuration file.
void unloadLibraries()
Close library handler.
std::vector< std::string > path_
std::shared_ptr< DynamicLibrary > lib_
Pointer to the library object.