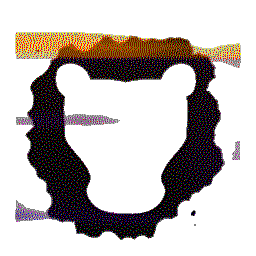 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #ifndef LEOSACEXCEPTION_HPP
27 #define LEOSACEXCEPTION_HPP
53 virtual const char *
what() const noexcept final
83 #endif // LEOSACEXCEPTION_HPP
virtual const char * what() const noexcept final
virtual ~LEOSACException()
const Leosac::Tools::Stacktrace & trace() const
Get the stacktrace associated with this exception.
Exception class for DynLib related errors.
Exception class for Gpio related errors.
Exception class for modules.
Exception class for Device related errors.
Exception class for Signal related errors.
A base class for Leosac specific exception.
Leosac::Tools::Stacktrace trace_
Exception class for filesystem related errors.
Exception class for Script related errors.
LEOSACException(const std::string &message)
Exception class for Core related errors.