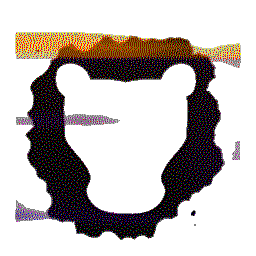 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
51 static std::string
getCWD();
60 const std::string &extension = std::string());
67 static std::string
stripPath(
const std::string &filename);
74 static std::string
readAll(
const std::string &path);
80 static bool fileExists(
const std::string &path);
90 std::ifstream file(path);
94 throw(
FsException(
"could not open \'" + path +
'\''));
106 template <
typename T>
109 std::ofstream file(path);
112 throw(
FsException(
"could not open " + path +
'\''));
This is the header file for a generated source file, GitSHA1.cpp.
Exception class for filesystem related errors.