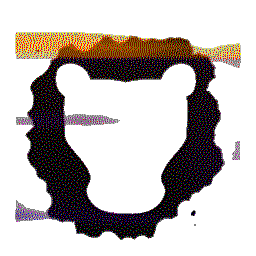 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include "boost/property_tree/ptree.hpp"
31 #include <zmqpp/actor.hpp>
96 mutable std::shared_ptr<DynamicLibrary>
lib_;
101 mutable std::unique_ptr<zmqpp::actor>
actor_;
148 bool loadModule(
const std::string &module_name);
163 bool has_module(
const std::string &name)
const;
bool operator<(const ModuleInfo &o) const
std::unique_ptr< zmqpp::actor > actor_
Actor object that runs the module code.
That class helps manage the configuration for the application and its module.
ModuleManager(zmqpp::context &ctx, Leosac::Kernel &k)
Construct the module manager.
A second module manager that loads "ZMQ aware" module – modules that talks to the application through...
void initModules()
Actually call the init_module() function of each library we loaded.
Leosac::CoreUtilsPtr core_utils_
This is the header file for a generated source file, GitSHA1.cpp.
ModuleInfo * find_module_by_name(const std::string &name) const
std::vector< std::string > modules_names() const
Returns the list of the name of the loaded modules.
const std::vector< std::string > & get_module_path() const
Return the list of paths where we search for module.
std::shared_ptr< DynamicLibrary > load_library_file(const std::string &full_path)
This will load (actually calling dlopen()) the library file located at full_path.
ModuleInfo(const Leosac::ConfigManager &cfg)
const Leosac::ConfigManager & cfg_
bool initModule(const std::string &name)
Attempt to find a module using its name, then load it.
Leosac::ConfigManager & config_manager_
std::set< ModuleInfo > modules_
Internal helper struct that store informations related to module that are useful to the module manage...
ModuleInfo & operator=(const ModuleInfo &)=delete
void stopModules(bool soft=false)
Opposite of init module.
void addToPath(const std::string &dir)
Add a directory to a path.
bool has_module(const std::string &name) const
Do we have some informations about the module "name".
bool loadModule(const std::string &module_name)
Search the path and load a module based on a property tree for this module.
std::shared_ptr< CoreUtils > CoreUtilsPtr
bool stopModule(const std::string &name)
Stop a module by name and remove its config info from the config manager.
std::string name_
Name of the module, as specified in the configuration file.
void unloadLibraries()
Close library handler.
std::vector< std::string > path_
std::shared_ptr< DynamicLibrary > lib_
Pointer to the library object.