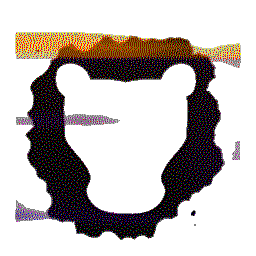 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #ifndef DYNAMICLIBRARY_HPP
27 #define DYNAMICLIBRARY_HPP
77 void *
getSymbol(
const std::string &symbol);
89 #endif // DYNAMICLIBRARY_HPP
void * getSymbol(const std::string &symbol)
Lookup a symbol by name and return a pointer to it.
const std::string & getFilePath() const
Returns the full path from which the library was loaded.
DynamicLibrary(const std::string &file)
Construct a dynamic library wrapper for the shared object referenced by name.
DynamicLibrary & operator=(const DynamicLibrary &other)=delete
Wraps a dynamic library handler and provide methods to interact with it.
void open(RelocationMode mode=RelocationMode::Lazy)
Attempts to open the shared library file so that we can access its symbols.
~DynamicLibrary()=default
void close()
Close the already opened library handler.