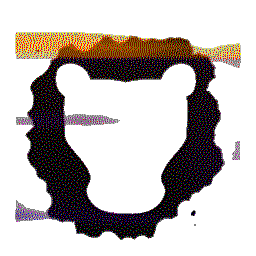 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
39 if (!(
_handle = dlopen(
_file.c_str(),
static_cast<int>(mode) | RTLD_NODELETE)))
41 if ((err = dlerror()))
59 sym = dlsym(
_handle, symbol.c_str());
60 if ((err = dlerror()))
void * getSymbol(const std::string &symbol)
Lookup a symbol by name and return a pointer to it.
const std::string & getFilePath() const
Returns the full path from which the library was loaded.
Exception class for DynLib related errors.
DynamicLibrary(const std::string &file)
Construct a dynamic library wrapper for the shared object referenced by name.
void open(RelocationMode mode=RelocationMode::Lazy)
Attempts to open the shared library file so that we can access its symbols.
void close()
Close the already opened library handler.