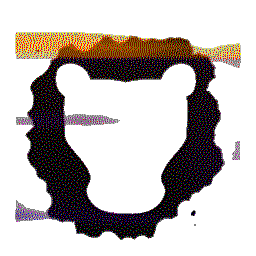 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
46 Config(
const std::string &config_target,
const std::string &config_entry,
63 const std::string &config_entry,
bool not_found)
65 using namespace Colorize;
68 return "Missing configuration entry for " +
green(config_target) +
": " +
73 return "Invalid configuration entry for " +
green(config_target) +
": " +
80 using namespace Colorize;
81 return "Failed to parse configuration file " +
underline(
green(filename));
91 :
LEOSACException(
"Configuration error in file {" + file +
"}: " + message)
virtual ~ConfigException()
std::string underline(const T &in)
This is the header file for a generated source file, GitSHA1.cpp.
Exception class for LEOSAC Project related errors.
A base class for Leosac specific exception.
ConfigException(const std::string &file, const std::string &message)
static std::string build_message(const std::string &config_target, const std::string &config_entry, bool not_found)
std::string green(const T &in)
std::string red(const T &in)
Config(const std::string &config_target, const std::string &config_entry, bool not_found)
Construct a config exception for when we failed to load a property.
An exception related to configuration.
static std::string build_message(const std::string &filename)
Config(const std::string &filename)
Create a config exception when we failed to parse a configuration file.