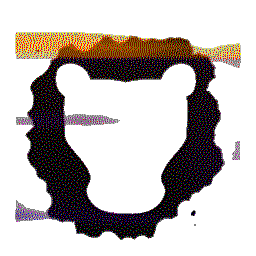 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <boost/property_tree/ptree.hpp>
29 #include <zmqpp/zmqpp.hpp>
96 const boost::property_tree::ptree &cfg);
101 bool has_config(
const std::string &module)
const;
106 const boost::property_tree::ptree &
load_config(
const std::string &module)
const;
127 const boost::property_tree::ptree &
kconfig()
const;
132 boost::property_tree::ptree &
kconfig();
140 void set_kconfig(
const boost::property_tree::ptree &new_cfg);
193 zmqpp::message &
operator>>(zmqpp::message &msg,
196 zmqpp::message &
operator<<(zmqpp::message &msg,
const boost::property_tree::ptree & load_config(const std::string &module) const
Return the stored configuration for a given module.
const std::string & instance_name() const
Return the name of the instanced assigned in the configuration file.
zmqpp::message & operator>>(zmqpp::message &msg, Leosac::ConfigManager::ConfigFormat &fmt)
That class helps manage the configuration for the application and its module.
ConfigManager & operator=(const ConfigManager &)=delete
ConfigFormat
This enum is used internally, when core request module configuration.
const boost::property_tree::ptree & kconfig() const
Return const & on the general config tree.
bool store_config(const std::string &module, const boost::property_tree::ptree &cfg)
Store the configuration tree for a module.
ConfigManager(const boost::property_tree::ptree &cfg)
Construct the Configuration Manager from a property tree.
void set_kconfig(const boost::property_tree::ptree &new_cfg)
Update Leosac's core config ptree.
std::string instance_name_
This is the header file for a generated source file, GitSHA1.cpp.
boost::property_tree::ptree get_general_config() const
Extract the current "general configuration".
std::map< std::string, boost::property_tree::ptree > modules_configs_
Maps a module's name to a property tree object.
Exception class for LEOSAC Project related errors.
bool has_config(const std::string &module) const
Do we have config information for the module.
void incr_version()
Increment by 1 the current version number.
boost::property_tree::ptree get_exportable_general_config() const
Return the property_tree of item inside the <kernel> tag (except <modules>`) that are marked exportab...
uint64_t config_version() const
Return the current configuration version.
std::list< std::string > get_non_importable_modules() const
Returns a list of module name that should not be imported.
bool is_module_importable(const std::string &) const
Helper around get_non_importable_modules()
boost::property_tree::ptree kernel_config_
Property tree for general configuration.
virtual ~ConfigManager()=default
bool remove_config(const std::string &module)
Remove the config entry for the module named module.
boost::property_tree::ptree get_application_config()
Retrieve the (current, running) configuration from the application and its modules.
zmqpp::message & operator<<(zmqpp::message &msg, const Leosac::ConfigManager::ConfigFormat &fmt)