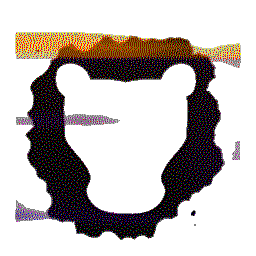 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
27 #include "tools/Schedule_odb.h"
49 std::vector<CRUDResourceHandler::ActionActionParam>
51 const json &req)
const
53 std::vector<CRUDResourceHandler::ActionActionParam> ret;
59 catch (
const json::out_of_range &e)
85 odb::transaction t(db->begin());
91 db->persist(schedule);
112 static void include_schedule_mapping_infos(
json &dest,
116 ASSERT_LOG(dest.is_array(),
"Destination is not array.");
117 for (
const auto &mapping : schedule.
mapping())
128 using Result = odb::result<Tools::Schedule>;
130 odb::transaction t(db->begin());
133 rep[
"included"] = json::array();
141 include_schedule_mapping_infos(rep[
"included"], *schedule,
147 rep[
"data"] = json::array();
148 for (
const auto &schedule : result)
152 include_schedule_mapping_infos(rep[
"included"], schedule,
163 odb::transaction t(db->begin());
166 rep[
"included"] = json::array();
179 for (
const auto &mapping : schedule->
mapping())
183 for (
const auto &lazy_weak_door : mapping->doors())
185 auto lazy_door =
LEOSAC_ENFORCE(lazy_weak_door.load(),
"Failed to load");
188 door_event->finalize();
194 for (
const auto &json_mapping : req.at(
"mapping"))
197 std::make_shared<Tools::ScheduleMapping>();
200 db->persist(mapping);
203 include_schedule_mapping_infos(rep[
"included"], *schedule,
security_context());
205 db->update(assert_cast<Tools::SchedulePtr>(schedule));
220 odb::transaction t(db->begin());
233 for (
const auto &mapping : schedule->
mapping())
virtual std::vector< ActionActionParam > required_permission(Verb verb, const json &req) const override
static SecurityContext & instance()
virtual boost::optional< json > read_impl(const json &req) override
ScheduleCRUD(RequestContext ctx)
std::unique_ptr< CRUDResourceHandler > CRUDResourceHandlerUPtr
#define ASSERT_LOG(cond, msg)
Base CRUD handler for use within the websocket module.
virtual boost::optional< json > update_impl(const json &req) override
Update a schedule object.
static IDoorEventPtr DoorEvent(const DBPtr &database, Auth::IDoorPtr target_door, IAuditEntryPtr parent)
std::shared_ptr< odb::database > DBPtr
All modules that provides features to Leosac shall be in this namespace.
@ MAPPING_MAY_HAVE_CHANGED
This event is linked to door.
Tools::ScheduleId schedule_id
This is the header file for a generated source file, GitSHA1.cpp.
virtual boost::optional< json > delete_impl(const json &req) override
std::shared_ptr< IScheduleEvent > IScheduleEventPtr
Audit::IAuditEntryPtr audit
The initial audit trail for the request.
Holds valuable pointer to provide context to a request.
static CRUDResourceHandlerUPtr instanciate(RequestContext)
static IScheduleEventPtr ScheduleEvent(const DBPtr &database, Tools::ISchedulePtr target_sched, IAuditEntryPtr parent)
#define LEOSAC_ENFORCE(cond,...)
Similar to enforce, except that it will throw a LEOSACException.
virtual boost::optional< json > create_impl(const json &req) override
Create a new schedule.
A SecurityContext is used to query permission while doing an operation.
odb::result< Tools::LogEntry > Result
virtual UserSecurityContext & security_context() const override
Helper function that returns the security context.