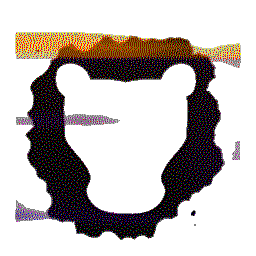 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
38 class ISchedule :
public std::enable_shared_from_this<ISchedule>
48 virtual const std::string &
name()
const = 0;
50 virtual void name(
const std::string &) = 0;
60 is_in_schedule(
const std::chrono::system_clock::time_point &tp)
const = 0;
76 virtual std::vector<SingleTimeFrame>
timeframes()
const = 0;
82 virtual std::vector<Tools::ScheduleMappingPtr>
mapping()
const = 0;
This is the header file for a generated source file, GitSHA1.cpp.