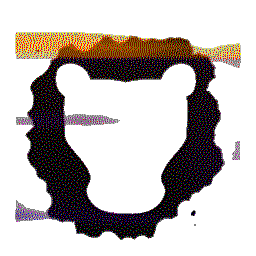 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "core/auth/Door_odb.h"
23 #include "core/auth/User_odb.h"
40 {
"type",
"schedule-mapping"},
43 json users = json::array();
44 for (
const auto &user : in.
users())
46 json json_user = {{
"id", user.object_id()}, {
"type",
"user"}};
47 users.push_back(json_user);
50 json groups = json::array();
51 for (
const auto &group : in.
groups())
53 json json_group = {{
"id", group.object_id()}, {
"type",
"group"}};
54 groups.push_back(json_group);
57 json creds = json::array();
63 {
"id", cred.object_id()},
65 creds.push_back(json_cred);
68 json doors = json::array();
69 for (
const auto &door : in.
doors())
71 json json_door = {{
"id", door.object_id()}, {
"type",
"door"}};
72 doors.push_back(json_door);
75 serialized[
"relationships"][
"users"] = {{
"data", users}};
76 serialized[
"relationships"][
"groups"] = {{
"data", groups}};
77 serialized[
"relationships"][
"credentials"] = {{
"data", creds}};
78 serialized[
"relationships"][
"doors"] = {{
"data", doors}};
89 using namespace JSONUtil;
92 auto group_ids = in.at(
"groups");
94 for (
const auto &group_id : group_ids)
100 auto user_ids = in.at(
"users");
102 for (
const auto &user_id : user_ids)
108 auto credential_ids = in.at(
"credentials");
110 for (
const auto &credential_id : credential_ids)
117 auto door_ids = in.at(
"doors");
119 for (
const auto &door_id : door_ids)
134 const std::string &in,
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
odb::lazy_shared_ptr< Group > GroupLPtr
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
Provides various database-related services to consumer.
This is the header file for a generated source file, GitSHA1.cpp.
odb::lazy_shared_ptr< User > UserLPtr
static std::string type_name(const Cred::ICredential &in)
Returns the "type-name" of the credential.
unsigned long CredentialId
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
odb::lazy_shared_ptr< Credential > CredentialLPtr
odb::lazy_shared_ptr< Door > DoorLPtr
A SecurityContext is used to query permission while doing an operation.