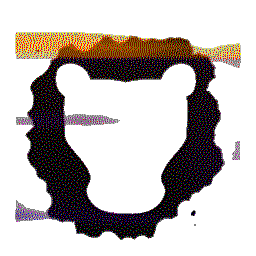 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <boost/algorithm/string.hpp>
34 json timeframes = json::array();
38 std::string start_time =
39 std::to_string(tf.start_hour) +
':' + std::to_string(tf.start_min);
40 std::string end_time =
41 std::to_string(tf.end_hour) +
':' + std::to_string(tf.end_min);
42 timeframes.push_back({{
"id", tf_pos},
43 {
"start-time", start_time},
44 {
"end-time", end_time},
49 json json_mappings = json::array();
50 for (
const auto &mapping : in.
mapping())
52 json json_mapping = {{
"id", mapping->id()}, {
"type",
"schedule-mapping"}};
53 json_mappings.push_back(json_mapping);
56 json serialized = {{
"id", in.
id()},
62 {
"timeframes", timeframes}}},
63 {
"relationships", {{
"mapping", {{
"data", json_mappings}}}}}};
71 static std::pair<int, int> parse_time(
const std::string &tm)
73 std::vector<std::string> splitted;
74 boost::algorithm::split(splitted, tm, boost::algorithm::is_any_of(
":"),
75 boost::algorithm::token_compress_on);
76 if (splitted.size() != 2)
78 BUILD_STR(
"Timeframe start or end time is invalid: " << tm));
80 return std::make_pair(std::stoi(splitted[0]), std::stoi(splitted[1]));
92 for (
const auto &tf_json : in.at(
"timeframes"))
94 std::pair<int, int> start_time = parse_time(tf_json.at(
"start-time"));
95 std::pair<int, int> end_time = parse_time(tf_json.at(
"end-time"));
97 SingleTimeFrame tf(tf_json.at(
"day"), start_time.first, start_time.second,
98 end_time.first, end_time.second);
110 const std::string &in,
Add a few useful extraction functions.
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
#define BUILD_STR(param)
Internal macro.
This is the header file for a generated source file, GitSHA1.cpp.
A base class for Leosac specific exception.
A SecurityContext is used to query permission while doing an operation.