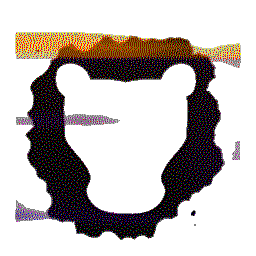 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
33 , sock_(ctx,
zmqpp::socket_type::rep)
35 , direction_(direction)
36 , initial_value_(initial_value)
38 , path_cfg_(module.general_config())
39 , next_update_time_(std::chrono::system_clock::time_point::max())
41 sock_.bind(
"inproc://" + name);
55 file_fd_ = open(full_path.c_str(), O_RDONLY | O_NONBLOCK);
72 ERROR(
"Error while resetting gpio state: " << e.
what());
86 ERROR(
"Error while unexporting GPIO: " << e.
what());
114 zmqpp::message_t msg;
122 else if (frame1 ==
"OFF")
124 else if (frame1 ==
"TOGGLE")
126 sock_.send(ok ?
"OK" :
"KO");
135 if (msg && msg->remaining() == 1)
143 std::chrono::system_clock::now() + std::chrono::milliseconds(duration);
147 WARN(
"Called with unexpected number of arguments: " << msg->remaining());
174 std::array<char, 64> buffer;
179 ret = ::read(
file_fd_, &buffer[0], buffer.size());
180 ASSERT_LOG(ret >= 0,
"Read failed on GPIO pin.");
181 ret = ::lseek(
file_fd_, 0, SEEK_SET);
182 ASSERT_LOG(ret >= 0,
"Lseek failed on GPIO pin.");
192 zmqpp::poller::poll_pri);
202 DEBUG(
"Turning off SysFsGPIO pin.");
void update()
Update the PIN.
std::string direction_path(int pin_no) const
Compute the absolute path the "direction" file for pin_no.
virtual const char * what() const noexcept final
std::chrono::system_clock::time_point next_update() const
This method shall returns the time point at which we want to be updated.
SysFsGpioPin(zmqpp::context &ctx, const std::string &name, int gpio_no, Direction direction, InterruptMode interrupt_mode, bool initial_value, SysFsGpioModule &module)
void handle_interrupt()
Interrupt happened for this GPIO ping.
void set_direction(Direction dir)
Write direction to the direction file.
#define ASSERT_LOG(cond, msg)
const bool initial_value_
Initial value of the PIN.
void publish_on_bus(zmqpp::message &msg)
Write the message eon the bus.
bool read_value()
Read value from filesystem.
bool toggle()
Read to sysfs and then write the opposite value.
unix filesystem helper functions
std::string value_path(int pin_no) const
Compute the absolute path the "value" file for pin_no.
const SysFsGpioConfig & general_config() const
Retrieve a reference to the config object.
zmqpp::socket sock_
listen to command from other component.
void handle_message()
The SysFsGpioModule will register this method so its called when a message is ready on the pin socket...
Namespace for the module that implements GPIO support using the Linux Kernel sysfs interface.
void set_interrupt(InterruptMode mode)
Write interrupt mode to the edge file.
std::chrono::system_clock::time_point next_update_time_
Time point of next wished update.
std::string edge_path(int pin_no) const
Compute the absolute path the "edge" file for pin_no.
bool turn_on(zmqpp::message *msg=nullptr)
Write to sysfs to turn the gpio on.
int gpio_no_
Number of the GPIO.
bool turn_off()
Write to sysfs to turn the gpio on.
Handle GPIO management over sysfs.
void register_sockets(zmqpp::reactor *reactor)
Register own socket to the module's reactor.
int file_fd_
File descriptor of the GPIO in sysfs.
const SysFsGpioConfig & path_cfg_
SysFsGpioModule & module_
Reference to the module.
const Direction direction_
Direction of the PIN.
const std::string & unexport_path() const
Returns the absolute path to the "unexport" sysfs file.