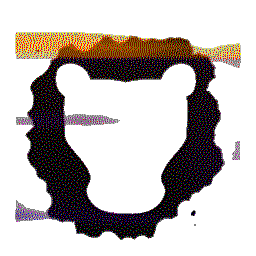 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <boost/algorithm/string.hpp>
38 auto aliases_cfg = cfg.get_child(
"aliases");
39 for (
auto alias : aliases_cfg)
41 if (alias.first ==
"default")
47 int pin_no = std::stoi(alias.first);
51 using namespace Colorize;
52 INFO(
"SysFsGpio Path Configuration:"
78 return boost::replace_all_copy(
80 boost::replace_all_copy(
default_aliases_,
"__NO__", std::to_string(pin_no)));
88 return boost::replace_all_copy(
90 boost::replace_all_copy(
default_aliases_,
"__NO__", std::to_string(pin_no)));
98 return boost::replace_all_copy(
100 boost::replace_all_copy(
default_aliases_,
"__NO__", std::to_string(pin_no)));
std::string direction_path(int pin_no) const
Compute the absolute path the "direction" file for pin_no.
std::string default_aliases_
Default aliases rule, as defined in configuration.
std::string cfg_edge_path_
Absolute path to the "edge" file.
std::map< int, std::string > pin_aliases_
Maps pin number to file identifier.
std::string cfg_export_path_
Absolute path of the "export" sysfs file.
std::string value_path(int pin_no) const
Compute the absolute path the "value" file for pin_no.
SysFsGpioConfig(const boost::property_tree::ptree &cfg)
Construct the general config object from the configuration tree.
Namespace for the module that implements GPIO support using the Linux Kernel sysfs interface.
std::string edge_path(int pin_no) const
Compute the absolute path the "edge" file for pin_no.
std::string green(const T &in)
std::string cfg_direction_path_
Absolute path to the "direction" file.
std::string cfg_value_path_
Absolute path of the "value file" sysfs file: use __PLACEHOLDER__ as a placeholder for the PIN identi...
std::string cfg_unexport_path_
Absolute path of the "unexport" sysfs file.
const std::string & export_path() const
Returns the absolute path to the "export" sysfs file.
const std::string & unexport_path() const
Returns the absolute path to the "unexport" sysfs file.