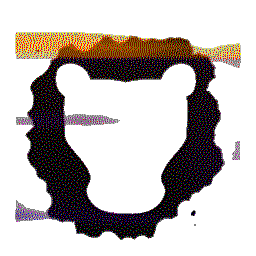 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <zmqpp/zmqpp.hpp>
32 class SysFsGpioModule;
33 class SysFsGpioConfig;
54 SysFsGpioPin(zmqpp::context &ctx,
const std::string &name,
int gpio_no,
77 std::chrono::system_clock::time_point
next_update()
const;
102 bool turn_on(zmqpp::message *msg =
nullptr);
void update()
Update the PIN.
std::chrono::system_clock::time_point next_update() const
This method shall returns the time point at which we want to be updated.
SysFsGpioPin(zmqpp::context &ctx, const std::string &name, int gpio_no, Direction direction, InterruptMode interrupt_mode, bool initial_value, SysFsGpioModule &module)
void handle_interrupt()
Interrupt happened for this GPIO ping.
SysFsGpioPin & operator=(const SysFsGpioPin &)=delete
void set_direction(Direction dir)
Write direction to the direction file.
const bool initial_value_
Initial value of the PIN.
bool read_value()
Read value from filesystem.
bool toggle()
Read to sysfs and then write the opposite value.
Internal configuration helper for sysfsgpio module.
This is the header file for a generated source file, GitSHA1.cpp.
zmqpp::socket sock_
listen to command from other component.
void handle_message()
The SysFsGpioModule will register this method so its called when a message is ready on the pin socket...
void set_interrupt(InterruptMode mode)
Write interrupt mode to the edge file.
std::chrono::system_clock::time_point next_update_time_
Time point of next wished update.
bool turn_on(zmqpp::message *msg=nullptr)
Write to sysfs to turn the gpio on.
int gpio_no_
Number of the GPIO.
bool turn_off()
Write to sysfs to turn the gpio on.
Handle GPIO management over sysfs.
void register_sockets(zmqpp::reactor *reactor)
Register own socket to the module's reactor.
Hardware::GPIO::Direction Direction
int file_fd_
File descriptor of the GPIO in sysfs.
This is a implementation class.
const SysFsGpioConfig & path_cfg_
SysFsGpioModule & module_
Reference to the module.
const Direction direction_
Direction of the PIN.