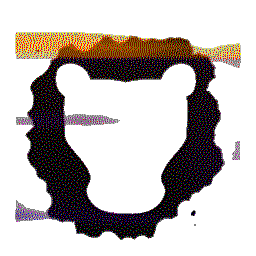 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 bool sync_general_config,
bool autocommit)
34 , fetch_task_(fetch_task)
35 , sync_general_config_(sync_general_config)
36 , autocommit_(autocommit)
46 WARN(
"Parent task (fetching config data) failed. Aborting SyncConfig task.");
55 catch (
const std::exception &e)
57 ERROR(
"SyncConfig task had a problem " << e.what());
71 INFO(
"Also syncing general configuration.");
80 DEBUG(
"Handling module {" << name <<
"}");
83 INFO(
"Updating config for {" << name <<
"}");
87 for (
const std::pair<std::string, std::string> &file_info :
90 INFO(
"Writing additional config file " << file_info.first);
91 std::ofstream of(file_info.first);
92 of << file_info.second;
98 DEBUG(
"Apparently {" << name
99 <<
"} is immutable (declared in <no_import>)");
117 DEBUG(
"Reloading config from backup for " << name);
122 DEBUG(
"Not reload config from backup for " << name);
135 ERROR(
"Cannot load module " << name <<
"after synchronisation.");
143 INFO(
"Saving configuration to disk after synchronization.");
uint64_t remote_version() const
const boost::property_tree::ptree & load_config(const std::string &module) const
Return the stored configuration for a given module.
void restart_later()
Set the running_ and want_restart flag so that leosac will restart in the next main loop iteration.
bool sync_general_config_
This class provides an API to collect the configuration of a remote Leosac unit.
That class helps manage the configuration for the application and its module.
const boost::property_tree::ptree & module_config(const std::string &name) const
Returns the configuration for one specific module, identified by name.
SyncConfig(Kernel &kref, FetchRemoteConfigPtr fetch_task, bool sync_general_config, bool autocommit)
void initModules()
Actually call the init_module() function of each library we loaded.
bool store_config(const std::string &module, const boost::property_tree::ptree &cfg)
Store the configuration tree for a module.
void set_kconfig(const boost::property_tree::ptree &new_cfg)
Update Leosac's core config ptree.
const boost::property_tree::ptree & general_config() const
Returns the tree for the general configuration option.
This is the header file for a generated source file, GitSHA1.cpp.
std::vector< std::string > modules_names() const
Returns the list of the name of the loaded modules.
bool has_config(const std::string &module) const
Do we have config information for the module.
bool save_config()
Save the current configuration to its original file if autosave is enabled.
const std::list< std::string > & modules_list() const noexcept
Return the list of modules that are loaded on the remote host.
FetchRemoteConfigPtr fetch_task_
The task that fetch the data.
uint64_t config_version() const
Return the current configuration version.
std::list< std::string > get_non_importable_modules() const
Returns a list of module name that should not be imported.
bool is_module_importable(const std::string &) const
Helper around get_non_importable_modules()
void stopModules(bool soft=false)
Opposite of init module.
const FileNameContentList & additional_files(const std::string module) const
bool loadModule(const std::string &module_name)
Search the path and load a module based on a property tree for this module.
std::shared_ptr< FetchRemoteConfig > FetchRemoteConfigPtr
CoreUtilsPtr core_utils()
Returns a (smart) pointer to the core utils: some thread-safe utilities.
const std::string & get_guid() const
const ModuleManager & module_manager() const
Returns a reference to the module manager object (const version).
ConfigManager & config_manager()
Retrieve a reference to the configuration manager object.