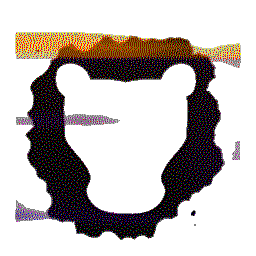 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
29 #include <zmqpp/curve.hpp>
34 std::string
const &remote_endpoint,
35 std::string
const &remote_pk)
36 : remote_endpoint_(remote_endpoint)
37 , remote_pk_(remote_pk)
38 , sock_(ctx,
zmqpp::socket_type::dealer)
43 auto kp = zmqpp::curve::generate_keypair();
44 sock_.set(zmqpp::socket_option::curve_secret_key, kp.secret_key);
45 sock_.set(zmqpp::socket_option::curve_public_key, kp.public_key);
46 sock_.set(zmqpp::socket_option::curve_server_key, remote_pk);
47 sock_.set(zmqpp::socket_option::linger, 0);
52 static bool warn_and_set_error(std::string *error_str,
const std::string &msg)
67 sock_.connect(remote_endpoint_);
68 if (!fetch_remote_config_version(remote_version_))
69 return warn_and_set_error(error_str,
70 "Cannot retrieve remote config version.");
72 if (!fetch_general_config())
73 return warn_and_set_error(
75 build_str(
"Error fetching general configuration of remote Leosac (",
76 remote_endpoint_,
")"));
78 if (!fetch_module_list())
79 return warn_and_set_error(
81 build_str(
"Error fetching module list from remote Leosac (",
82 remote_endpoint_,
")"));
84 if (!fetch_modules_config())
85 return warn_and_set_error(
88 "Error fetching modules configuration from remote Leosac (",
89 remote_endpoint_,
")"));
92 if (!fetch_remote_config_version(version2))
93 return warn_and_set_error(
94 error_str,
build_str(
"Cannot retrieve remote config version."));
95 if (version2 != remote_version_)
96 return warn_and_set_error(error_str,
97 build_str(
"Looks like configuration changed "
98 "while we were retrieving it."));
102 catch (std::exception &e)
106 *error_str = std::string(
"Exception occured: ") + e.what();
122 if (msg.remaining() == 2)
137 sock_.send(
"MODULE_LIST");
145 while (msg.remaining())
160 msg <<
"MODULE_CONFIG" << module_name
168 if (msg.remaining() < 3)
172 std::string config_str;
173 std::string recv_module_name;
175 msg >> result >> recv_module_name >> config_str;
176 assert(result ==
"OK");
177 assert(recv_module_name == module_name);
180 if (msg.remaining() % 2 != 0)
182 ERROR(
"Msg has " << msg.remaining()
183 <<
" remaining parts, but need a multiple of 2.");
186 while (msg.remaining())
188 std::string file_name;
189 std::string file_content;
191 msg >> file_name >> file_content;
193 std::make_pair(file_name, file_content));
228 const boost::property_tree::ptree &
236 throw std::runtime_error(
"Code is broken: module " + name +
237 " doesn't exist in this config map.");
251 throw std::runtime_error(
"Module doesn't exist here.");
256 auto task = std::make_shared<Tasks::GetRemoteConfigVersion>(
remote_endpoint_,
261 version = task->config_version_;
void print_exception(const std::exception &e, int level=0)
Recursively print the exception trace to std::cerr.
uint64_t remote_version() const
const boost::property_tree::ptree & module_config(const std::string &name) const
Returns the configuration for one specific module, identified by name.
bool fetch_remote_config_version(uint64_t &version)
Fetch the version of the remote configuration.
zmqpp::poller_t poller_
Poll on the socket.
bool fetch_module_config(const std::string &module_name)
Sends the MODULE_CONFIG command for the module whose name is module_name.
boost::property_tree::ptree general_config_
bool fetch_module_list()
Sends the MODULE_LIST command.
bool fetch_modules_config()
Fetch the conf for all modules (relying on fetch_module_config()).
bool fetch_general_config()
Send the GENERAL_CONFIG command to the remote, and wait for response.
ModuleAdditionalFiles additional_files_
const boost::property_tree::ptree & general_config() const
Returns the tree for the general configuration option.
This is the header file for a generated source file, GitSHA1.cpp.
std::string build_str(Args... args)
Use a stringstream to build a string that combine all arguments.
std::list< std::pair< std::string, std::string > > FileNameContentList
bool fetch_config(std::string *error_str) noexcept
Fetch the complete remote configuration.
const std::list< std::string > & modules_list() const noexcept
Return the list of modules that are loaded on the remote host.
std::map< std::string, boost::property_tree::ptree > ModuleConfigMap
std::string remote_endpoint_
ModuleConfigMap config_map_
Map module name to their config tree.
RemoteConfigCollector(zmqpp::context_t &ctx, const std::string &remote_endpoint, const std::string &remote_pk)
Construct a new RemoteConfigCollector, this object will work for a one-time config collection.
const FileNameContentList & additional_files(const std::string module) const
std::list< std::string > module_list_
const ModuleConfigMap & modules_config() const noexcept
Returns a reference to modules and their configuration.