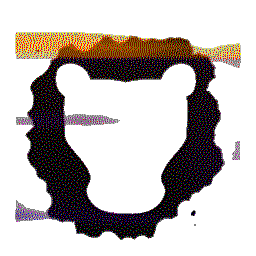 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include <boost/property_tree/ptree.hpp>
27 #include <zmqpp/zmqpp.hpp>
66 const std::string &remote_pk);
98 const std::list<std::string> &
modules_list() const noexcept;
108 const boost::property_tree::ptree &
module_config(const std::
string &name) const;
uint64_t remote_version() const
This class provides an API to collect the configuration of a remote Leosac unit.
RemoteConfigCollector & operator=(const RemoteConfigCollector &)=delete
const boost::property_tree::ptree & module_config(const std::string &name) const
Returns the configuration for one specific module, identified by name.
bool fetch_remote_config_version(uint64_t &version)
Fetch the version of the remote configuration.
zmqpp::poller_t poller_
Poll on the socket.
bool fetch_module_config(const std::string &module_name)
Sends the MODULE_CONFIG command for the module whose name is module_name.
boost::property_tree::ptree general_config_
bool fetch_module_list()
Sends the MODULE_LIST command.
bool fetch_modules_config()
Fetch the conf for all modules (relying on fetch_module_config()).
bool fetch_general_config()
Send the GENERAL_CONFIG command to the remote, and wait for response.
ModuleAdditionalFiles additional_files_
const boost::property_tree::ptree & general_config() const
Returns the tree for the general configuration option.
This is the header file for a generated source file, GitSHA1.cpp.
std::map< std::string, FileNameContentList > ModuleAdditionalFiles
Map module name to a list of (file_name, file_content)
std::list< std::pair< std::string, std::string > > FileNameContentList
bool fetch_config(std::string *error_str) noexcept
Fetch the complete remote configuration.
const std::list< std::string > & modules_list() const noexcept
Return the list of modules that are loaded on the remote host.
std::map< std::string, boost::property_tree::ptree > ModuleConfigMap
std::string remote_endpoint_
ModuleConfigMap config_map_
Map module name to their config tree.
virtual ~RemoteConfigCollector()=default
RemoteConfigCollector(zmqpp::context_t &ctx, const std::string &remote_endpoint, const std::string &remote_pk)
Construct a new RemoteConfigCollector, this object will work for a one-time config collection.
const FileNameContentList & additional_files(const std::string module) const
list(APPEND CMAKE_MODULE_PATH ${CMAKE_SOURCE_DIR}/cmake) set(LEOSAC_BIN leosac) set(LEOSAC_LIB leosac_lib) set(LEOSAC_SRCS main.cpp) set(LEOSAC_LIB_SRCS core/kernel.cpp core/CoreAPI.cpp core/config/ConfigManager.cpp core/config/RemoteConfigCollector.cpp core/config/ConfigChecker.cpp core/CoreUtils.cpp core/RemoteControl.cpp core/RemoteControlSecurity.cpp core/module_manager.cpp core/MessageBus.cpp core/Scheduler.cpp core/tasks/Task.cpp core/tasks/GenericTask.cpp core/netconfig/networkconfig.cpp core/auth/Auth.cpp core/auth/Group.cpp core/auth/ProfileMerger.cpp core/auth/SimpleAccessProfile.cpp core/auth/BaseAuthSource.cpp core/auth/AuthTarget.cpp core/auth/User.cpp core/auth/serializers/UserSerializer.cpp core/auth/serializers/GroupSerializer.cpp core/auth/serializers/UserGroupMembershipSerializer.cpp core/auth/serializers/DoorSerializer.cpp core/auth/serializers/AccessPointSerializer.cpp core/auth/serializers/ZoneSerializer.cpp core/auth/Token.cpp core/auth/UserGroupMembership.cpp core/auth/AuthSourceBuilder.cpp core/auth/ValidityInfo.cpp core/auth/Door.cpp core/auth/AccessPoint.cpp core/auth/AccessPointUpdate.cpp core/auth/AccessPointService.cpp core/auth/Zone.cpp core/credentials/Credential.cpp core/credentials/CredentialValidator.cpp core/credentials/RFIDCard.cpp core/credentials/PinCode.cpp core/credentials/RFIDCardPin.cpp core/credentials/serializers/CredentialSerializer.cpp core/credentials/serializers/RFIDCardSerializer.cpp core/credentials/serializers/PolymorphicCredentialSerializer.cpp core/credentials/serializers/PinCodeSerializer.cpp core/UserSecurityContext.cpp core/GetServiceRegistry.cpp dynlib/dynamiclibrary.cpp modules/AsioModule.cpp modules/BaseModule.cpp exception/ExceptionsTools.cpp exception/ModelException.cpp exception/EntityNotFound.cpp exception/PermissionDenied.cpp hardware/facades/FGPIO.cpp hardware/facades/FLED.cpp hardware/facades/FWiegandReader.cpp hardware/facades/FExternalServer.cpp hardware/Device.cpp hardware/GPIO.cpp hardware/RFIDReader.cpp hardware/Buzzer.cpp hardware/LED.cpp hardware/ExternalMessage.cpp hardware/ExternalServer.cpp hardware/HardwareService.cpp hardware/serializers/RFIDReaderSerializer.cpp hardware/serializers/GPIOSerializer.cpp hardware/serializers/DeviceSerializer.cpp hardware/serializers/LEDSerializer.cpp hardware/serializers/BuzzerSerializer.cpp hardware/serializers/ExternalMessageSerializer.cpp hardware/serializers/ExternalServerSerializer.cpp tools/runtimeoptions.cpp tools/signalhandler.cpp tools/unixshellscript.cpp tools/unixsyscall.cpp tools/unixfilewatcher.cpp tools/unixfs.cpp tools/version.cpp tools/Schedule.cpp tools/XmlPropertyTree.cpp tools/XmlScheduleLoader.cpp tools/ThreadUtils.cpp tools/GenGuid.cpp tools/PropertyTreeExtractor.cpp tools/log.cpp tools/DatabaseLogSink.cpp tools/ElapsedTimeCounter.cpp tools/XmlNodeNameEnforcer.cpp tools/Stacktrace.cpp tools/LogEntry.cpp tools/db/DBService.cpp tools/db/MultiplexedSession.cpp tools/db/MultiplexedTransaction.cpp tools/db/OptionalTransaction.cpp tools/db/Savepoint.cpp tools/scrypt/Random.cpp tools/scrypt/Scrypt.cpp tools/registry/ThreadLocalRegistry.cpp tools/registry/GlobalRegistry.cpp tools/JSONUtils.cpp tools/MyTime.cpp tools/SingleTimeFrame.cpp tools/serializers/ScheduleSerializer.cpp tools/serializers/ScheduleMappingSerializer.cpp tools/ScheduleMapping.cpp core/tasks/GetLocalConfigVersion.cpp core/tasks/GetRemoteConfigVersion.cpp core/tasks/FetchRemoteConfig.cpp core/tasks/SyncConfig.cpp core/tasks/RemoteControlAsyncResponse.cpp core/audit/AuditEntry.cpp core/audit/UserEvent.cpp core/audit/WSAPICall.cpp core/audit/AuditFactory.cpp core/audit/GroupEvent.cpp core/audit/UserGroupMembershipEvent.cpp core/audit/CredentialEvent.cpp core/audit/ScheduleEvent.cpp core/audit/DoorEvent.cpp core/audit/UpdateEvent.cpp core/audit/AccessPointEvent.cpp core/audit/AuditTracker.cpp core/audit/ZoneEvent.cpp core/audit/serializers/AuditSerializer.cpp core/audit/serializers/UserEventSerializer.cpp core/audit/serializers/PolymorphicAuditSerializer.cpp core/audit/serializers/WSAPICallSerializer.cpp core/audit/serializers/CredentialEventSerializer.cpp core/audit/serializers/GroupEventSerializer.cpp core/audit/serializers/ScheduleEventSerializer.cpp core/audit/serializers/DoorEventSerializer.cpp core/audit/serializers/UserGroupMembershipEventSerializer.cpp core/audit/serializers/AccessPointEventSerializer.cpp core/audit/serializers/ZoneEventSerializer.cpp core/update/UpdateService.cpp core/update/Update.cpp core/update/serializers/AccessPointUpdateSerializer.cpp core/update/serializers/UpdateSerializer.cpp core/update/serializers/UpdateDescriptorSerializer.cpp tools/db/PGSQLTracer.cpp tools/Visitor.cpp core/SecurityContext.cpp core/audit/serializers/UpdateEventSerializer.cpp hardware/serializers/BuzzerSerializer.cpp hardware/serializers/BuzzerSerializer.hpp) include(GetGitRevisionDescription) get_git_head_revision(GIT_REFSPEC GIT_SHA1) configure_file("$
std::list< std::string > module_list_
const ModuleConfigMap & modules_config() const noexcept
Returns a reference to modules and their configuration.