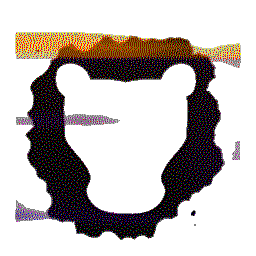 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include "core/auth/User_odb.h"
50 using Query = odb::query<Auth::User>;
52 odb::transaction t(db->begin());
53 json attributes = req.at(
"attributes");
56 new_user->username(attributes.at(
"username"));
57 if (db->query_one<
Auth::User>(Query::username == new_user->username()))
59 BUILD_STR(
"The username " << new_user->username()
60 <<
" is already in use."));
63 db->persist(new_user);
81 using Result = odb::result<Auth::User>;
83 odb::transaction t(db->begin());
96 rep[
"data"] = json::array();
97 for (
const auto &user : result)
99 rep[
"data"].push_back(
112 odb::transaction t(db->begin());
114 auto attributes = req.at(
"attributes");
123 bool enabled_status = user->validity().is_enabled();
127 if (enabled_status && !user->validity().is_enabled() &&
146 std::vector<CRUDResourceHandler::ActionActionParam>
149 std::vector<CRUDResourceHandler::ActionActionParam> ret;
156 catch (
const json::out_of_range &e)
static SecurityContext & instance()
#define BUILD_STR(param)
Internal macro.
static json serialize(const Auth::User &in, const SecurityContext &sc)
static CRUDResourceHandlerUPtr instanciate(RequestContext)
std::unique_ptr< CRUDResourceHandler > CRUDResourceHandlerUPtr
Base CRUD handler for use within the websocket module.
std::shared_ptr< User > UserPtr
virtual boost::optional< json > update_impl(const json &req) override
Update information about a given user.
std::shared_ptr< odb::database > DBPtr
All modules that provides features to Leosac shall be in this namespace.
odb::query< Tools::LogEntry > Query
This is the header file for a generated source file, GitSHA1.cpp.
std::shared_ptr< IUserEvent > IUserEventPtr
A base class for Leosac specific exception.
virtual boost::optional< json > delete_impl(const json &req) override
UserCRUD(RequestContext ctx)
virtual std::vector< ActionActionParam > required_permission(Verb verb, const json &req) const override
static void unserialize(Auth::User &out, const json &in, const SecurityContext &sc)
An exception class for general API error.
static IUserEventPtr UserEvent(const DBPtr &database, Auth::UserPtr target_user, IAuditEntryPtr parent)
virtual boost::optional< json > create_impl(const json &req) override
Audit::IAuditEntryPtr audit
The initial audit trail for the request.
virtual boost::optional< json > read_impl(const json &req) override
Retrieve information about a given user, or about all users.
Holds valuable pointer to provide context to a request.
odb::result< Tools::LogEntry > Result
static std::string serialize(const Auth::User &in, const SecurityContext &sc)
virtual UserSecurityContext & security_context() const override
Helper function that returns the security context.