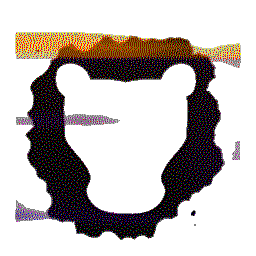 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include "core/credentials/Credential_odb.h"
27 #include "tools/Schedule_odb.h"
35 json memberships = {};
42 json group_info = {{
"id", membership->id()},
43 {
"type",
"user-group-membership"}};
44 memberships.push_back(group_info);
47 json credentials = {};
55 {
"id", cred.object_id()},
58 credentials.push_back(cred_info);
63 std::set<Tools::ScheduleId> schedule_ids;
67 auto loaded = mapping.load();
68 ASSERT_LOG(loaded,
"Cannot load. Need to investigate.");
69 schedule_ids.insert(loaded->schedule_id());
71 for (
const auto &
id : schedule_ids)
73 json sched_info = {{
"id",
id}, {
"type",
"schedule"}};
74 schedules.push_back(sched_info);
86 {
"rank",
static_cast<int>(user.
rank())},
92 {{
"memberships", {{
"data", memberships}}},
93 {
"credentials", {{
"data", credentials}}},
94 {
"schedules", {{
"data", schedules}}}}}};
100 serialized[
"attributes"][
"email"] = user.
email();
113 if (in.find(
"password") != in.end() && (*in.find(
"password")).is_string())
142 json tmp = json::parse(in);
const std::string & lastname() const
Holds classes relevant to the Authentication and Authorization subsystem.
Add a few useful extraction functions.
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
static json serialize(const Auth::User &in, const SecurityContext &sc)
virtual bool check_permission(Action a, const ActionParam &ap) const
Check for the permission to perform action a with parameters ap.
void password(const std::string &pw)
Set a new password for the user.
#define ASSERT_LOG(cond, msg)
static void unserialize(Auth::User &out, const std::string &in, const SecurityContext &sc)
UserRank rank() const
Get the global rank of the user.
bool is_enabled() const
Is the credential enabled ?
size_t odb_version() const
Auth::UserGroupMembershipId membership_id
Cred::CredentialId credential_id
odb::lazy_weak_ptr< Credential > CredentialLWPtr
This is the header file for a generated source file, GitSHA1.cpp.
const std::string & username() const noexcept
Get the username of this user.
const TimePoint & end() const
std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const
MembershipActionParam membership
UserId id() const noexcept
static std::string type_name(const Cred::ICredential &in)
Returns the "type-name" of the credential.
const UserGroupMembershipSet & group_memberships() const
Retrieve the UserGroupMembership that this user is involved with.
Auth::ValidityInfo extract_validity_with_default(const nlohmann::json &obj, const std::string &base_key, const Auth::ValidityInfo &def)
Extract fields representing a ValidityInfo object.
const TimePoint & start() const
static void unserialize(Auth::User &out, const json &in, const SecurityContext &sc)
@ USER_UPDATE_RANK
Editing rank means being able to become administrator.
const std::string & email() const
std::string format(const std::string &escape_code, const T &in)
Return a string containing the escape code, a string representation of T and the clear escape string.
const ValidityInfo & validity() const
@ USER_MANAGE_VALIDITY
Can we enable/disable the user or change its validity period ?
std::vector< Cred::CredentialLWPtr > lazy_credentials() const
A SecurityContext is used to query permission while doing an operation.
A simple class that stores (and can be queried for) the validity of some objects.
const std::string & firstname() const
static std::string serialize(const Auth::User &in, const SecurityContext &sc)