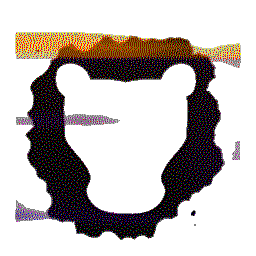 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include "core/update/Update_odb.h"
34 .track_foreign(backend));
38 boost::placeholders::_1, boost::placeholders::_2)
39 .track_foreign(backend));
43 boost::placeholders::_1, boost::placeholders::_2)
44 .track_foreign(backend));
48 boost::placeholders::_1, boost::placeholders::_2)
49 .track_foreign(backend));
60 for (
const auto &descriptor : descriptors)
76 std::vector<IUpdatePtr> updates;
77 using Query = odb::query<update::Update>;
81 for (
auto i(updates_odb.begin()); i != updates_odb.end(); ++i)
84 ASSERT_LOG(ptr,
"Loading failed, but object should already be loaded.");
85 updates.push_back(ptr);
An optional transaction is an object that behave like an odb::transaction if there is no currently ac...
void commit()
Commit the transaction, if there was no currently active transaction at the time of this object's cre...
virtual void cancel_update(IUpdatePtr u, const ExecutionContext &)=0
Cancel (not rollback) the pending update u.
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
virtual std::vector< UpdateDescriptorPtr > check_update()=0
Check for updates against arbitrary, module-owned object.
std::map< std::string, UpdateDescriptorPtr > published_descriptors_
#define ASSERT_LOG(cond, msg)
void cancel_update(IUpdatePtr update, const ExecutionContext &ec)
std::shared_ptr< IUpdate > IUpdatePtr
std::vector< IUpdatePtr > pending_updates()
Retrieve the list of pending updates.
odb::query< Tools::LogEntry > Query
Provides various database-related services to consumer.
CheckUpdateT check_update_sig_
CancelUpdateT cancel_update_sig_
std::shared_ptr< UpdateBackend > UpdateBackendPtr
This is the header file for a generated source file, GitSHA1.cpp.
void register_backend(UpdateBackendPtr backend)
Register a backend object.
An ExecutionContext is passed around to service so they have context about who is making the call and...
A base class for Leosac specific exception.
std::vector< UpdateDescriptorPtr > check_update()
std::string gen_uuid()
Generate a new UUID.
AckUpdateT ack_update_sig_
void ack_update(IUpdatePtr update, const ExecutionContext &ec)
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
virtual void ack_update(IUpdatePtr u, const ExecutionContext &)=0
Acknowledge the pending update u.
IUpdatePtr create_update(const std::string &update_descriptor_uuid, const ExecutionContext &ec)
Create an Update object corresponding to the update descriptor whose uuid is update_descriptor_uuid.
CreateUpdateT create_update_sig_
virtual IUpdatePtr create_update(const UpdateDescriptor &ud, const ExecutionContext &)=0
Create an update based on the UpdateDescriptor ud.