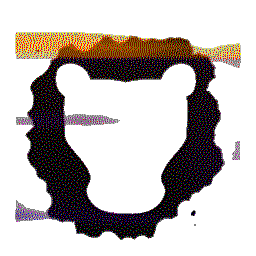 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
84 virtual std::vector<UpdateDescriptorPtr>
check_update() = 0;
166 boost::signals2::signal<std::vector<UpdateDescriptorPtr>(
void),
boost::signals2::signal< IUpdatePtr(const UpdateDescriptor &, const ExecutionContext &), AtMostOneCombiner< IUpdatePtr > > CreateUpdateT
virtual void cancel_update(IUpdatePtr u, const ExecutionContext &)=0
Cancel (not rollback) the pending update u.
boost::signals2::signal< void(IUpdatePtr, const ExecutionContext &)> AckUpdateT
boost::signals2::signal< std::vector< UpdateDescriptorPtr >(void), VectorAppenderCombiner< UpdateDescriptorPtr > > CheckUpdateT
virtual std::vector< UpdateDescriptorPtr > check_update()=0
Check for updates against arbitrary, module-owned object.
std::map< std::string, UpdateDescriptorPtr > published_descriptors_
void cancel_update(IUpdatePtr update, const ExecutionContext &ec)
std::shared_ptr< IUpdate > IUpdatePtr
Describe an update that has yet to be done.
std::vector< IUpdatePtr > pending_updates()
Retrieve the list of pending updates.
CheckUpdateT check_update_sig_
CancelUpdateT cancel_update_sig_
std::shared_ptr< UpdateBackend > UpdateBackendPtr
This is the header file for a generated source file, GitSHA1.cpp.
void register_backend(UpdateBackendPtr backend)
Register a backend object.
An ExecutionContext is passed around to service so they have context about who is making the call and...
A boost::signals2 combiner that makes sure that at most one slot returns a non-null pointer.
A shortname for the boost::signals2 namespace.
std::vector< UpdateDescriptorPtr > check_update()
virtual void implement_me_()=0
Just to make UpdateDescriptor abstract so module have to provide their own subclass.
AckUpdateT ack_update_sig_
Provides dynamic serializers management for a given object's hierarchy.
void ack_update(IUpdatePtr update, const ExecutionContext &ec)
This service provides various update management utilities.
virtual void ack_update(IUpdatePtr u, const ExecutionContext &)=0
Acknowledge the pending update u.
virtual ~UpdateDescriptor()=default
IUpdatePtr create_update(const std::string &update_descriptor_uuid, const ExecutionContext &ec)
Create an Update object corresponding to the update descriptor whose uuid is update_descriptor_uuid.
CreateUpdateT create_update_sig_
std::string source_module
virtual IUpdatePtr create_update(const UpdateDescriptor &ud, const ExecutionContext &)=0
Create an update based on the UpdateDescriptor ud.
boost::signals2::signal< void(IUpdatePtr, const ExecutionContext &)> CancelUpdateT