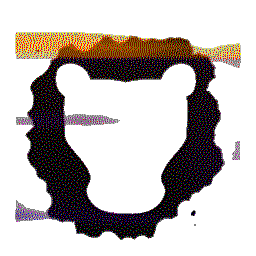 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include "core/auth/Token_odb.h"
28 #include "core/auth/User_odb.h"
36 #include <boost/algorithm/string.hpp>
37 #include <odb/object-result.hxx>
38 #include <odb/session.hxx>
51 ASSERT_LOG(token,
"nullptr passed when excepting non-null token.");
54 using namespace odb::core;
55 odb::transaction t(
server_.
db()->begin());
63 using namespace odb::core;
64 using query = odb::query<Auth::Token>;
67 transaction t(db->begin());
71 if (token && token->is_valid())
74 token->expire_in(std::chrono::minutes(20));
83 const std::string &password)
const
86 using namespace odb::core;
87 using query = odb::query<Auth::User>;
90 transaction t(db->begin());
92 auto username_lowercase = boost::algorithm::to_lower_copy(username);
94 db->query_one<
Auth::User>(query::username == username_lowercase);
95 if (user && user->verify_password(password))
99 auto token = std::make_shared<Auth::Token>(
gen_uuid(), user);
101 token->expire_in(std::chrono::minutes(20));
105 if (user->username() ==
"admin")
107 if (
const auto &mailer =
111 mail.
title =
"Admin Connected";
112 mail.
body =
"The user `admin` logged in !";
113 mailer->async_send_to_admin(mail);
125 const auto &validity = u.
validity();
126 if (!validity.is_enabled())
128 if (!validity.is_in_range())
130 BUILD_STR(
"This user account is not currently active."));
#define BUILD_STR(param)
Internal macro.
An authentication token used for authenticating a user against Leosac.
std::shared_ptr< Token > TokenPtr
void enforce_user_enabled(const Auth::User &u) const
Make sure the User u is authorized to log in.
Auth::TokenPtr authenticate_token(const std::string &token_str) const
Attempt to authenticate with an authentication token.
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
#define ASSERT_LOG(cond, msg)
std::shared_ptr< User > UserPtr
Acts like an odb::session, with the exception that it will save the current active session (if any) a...
Provide ODB magic to be able to store an Leosac::Audit::EventType (FlagSet) object.
Auth::TokenPtr authenticate_credentials(const std::string &username, const std::string &password) const
Attempt to authenticate with username/password credential and generate an authentication token.
All modules that provides features to Leosac shall be in this namespace.
This is the header file for a generated source file, GitSHA1.cpp.
WSServer & server_
Reference to the Websocket server.
A base class for Leosac specific exception.
The implementation class that runs the websocket server.
std::string gen_uuid()
Generate a new UUID.
void invalidate_token(Auth::TokenPtr token) const
Invalidate the authentication token, removing it from the database.
DBPtr db()
Retrieve database handle.
const ValidityInfo & validity() const