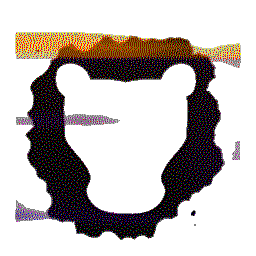 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
20 #include "core/auth/Group_odb.h"
21 #include "core/auth/User_odb.h"
26 #include <boost/algorithm/string.hpp>
30 static bool is_valid_username_character(
char c)
32 return isascii(c) && (isalnum(c) || c ==
'.' || c ==
'_' || c ==
'-');
58 ASSERT_LOG(is_valid_username_character(c),
"Invalid username.");
66 if (is_valid_username_character(c))
140 std::vector<uint8_t> vec(pw.begin(), pw.end());
148 std::vector<uint8_t> vec(pw.begin(), pw.end());
196 "Credential is already owned by someone else.");
198 cred->owner(shared_from_this());
199 credentials_.push_back(assert_cast<Cred::CredentialPtr>(cred));
const std::string & lastname() const
Holds classes relevant to the Authentication and Authorization subsystem.
bool is_valid() const
Check the validity status (enabled / disabled) of the user.
IAccessProfilePtr profile() const noexcept
IAccessProfilePtr profile_
static bool Verify(const std::vector< uint8_t > &in, const ScryptResult &expected)
Verify that the input in, when hashed, correspond to the expected ScryptResult.
#define ASSERT_LOG(cond, msg)
UserRank rank() const
Get the global rank of the user.
std::shared_ptr< IAccessProfile > IAccessProfilePtr
size_t odb_version() const
boost::optional< ScryptResult > password_
Exception class for LEOSAC Project related errors.
const std::string & username() const noexcept
Get the username of this user.
A base class for Leosac specific exception.
std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const
bool is_valid() const
Check that the current date is between validity start and end and make sure its enabled too.
UserId id() const noexcept
void schedule_mapping_added(const Tools::ScheduleMappingPtr &sched_mapping)
The user has been mapped by a schedule.
std::string password() const
Returns the password hash + salt (as stored in the database).
const UserGroupMembershipSet & group_memberships() const
Retrieve the UserGroupMembership that this user is involved with.
bool verify_password(const std::string &pw) const
Verify that the password pw is equal to the user's password.
std::shared_ptr< ICredential > ICredentialPtr
std::set< UserGroupMembershipPtr, UserGroupMembershipComparator > UserGroupMembershipSet
const std::string & email() const
const ValidityInfo & validity() const
std::vector< Cred::CredentialLWPtr > credentials_
std::vector< Tools::ScheduleMappingLWPtr > schedules_mapping_
ScheduleMapping object to which we are mapped directly (as user).
std::string username_
This is an (unique) identifier for the user.
std::vector< Cred::CredentialLWPtr > lazy_credentials() const
ValidityInfo validity_
A user can have the same validity than credentials.
static ScryptResult Hash(const std::vector< uint8_t > &in, const std::vector< uint8_t > &salt, const ScryptParam ¶m=default_)
Wrapper around low-level hash function.
A simple class that stores (and can be queried for) the validity of some objects.
const std::string & firstname() const
void add_credential(const Cred::ICredentialPtr &cred)
UserGroupMembershipSet membership_