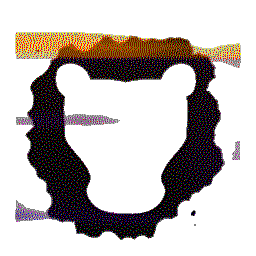 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
33 #include <boost/optional.hpp>
35 #include <type_traits>
36 #include <websocketpp/config/asio_no_tls.hpp>
37 #include <websocketpp/server.hpp>
38 #include <zmqpp/zmqpp.hpp>
71 using Server = websocketpp::server<websocketpp::config::asio>;
73 std::owner_less<websocketpp::connection_hdl>>;
75 void run(
const std::string &interface, uint16_t port);
96 const std::string &name);
145 void on_open(websocketpp::connection_hdl hdl);
147 void on_close(websocketpp::connection_hdl hdl);
166 void on_message(websocketpp::connection_hdl hdl, Server::message_ptr msg);
264 std::unique_ptr<boost::asio::io_service::work>
work_;
boost::optional< json > dispatch_request(APIPtr api_handle, const ClientMessage &in, Audit::IAuditEntryPtr)
Dispatch the request from a client, so that it is processed by the appropriate handler.
WSServer(WebSockAPIModule &module, DBPtr database)
std::map< std::string, MethodHandler::Factory > individual_handlers_
void on_open(websocketpp::connection_hdl hdl)
A message sent by the server to a client.
void register_crud_handler_external(const std::string &resource_name, CRUDResourceHandler::Factory factory)
Register a CRUD handler from an external thread.
std::map< std::string, CRUDResourceHandler::Factory > crud_handlers_
void unregister_handler(const std::string &name)
Remove an Asio based handler.
void run(const std::string &interface, uint16_t port)
std::shared_ptr< User > UserPtr
APIAuth & auth()
Retrieve the authentication helper.
This class is responsible for providing an API to manage authentication for Websocket client.
A message sent by a client to Leosac.
void clear_user_sessions(Auth::UserPtr user, APIPtr exception)
Deauthenticate all the connections of user, except the exception APISession.
std::shared_ptr< odb::database > DBPtr
CRUDResourceHandlerUPtr(*)(RequestContext) Factory
void send_message(websocketpp::connection_hdl hdl, const ServerMessage &msg)
Send a message over a connection.
websocketpp::server< websocketpp::config::asio > Server
std::shared_ptr< IAuditEntry > IAuditEntryPtr
void on_message(websocketpp::connection_hdl hdl, Server::message_ptr msg)
A websocket message has been received.
std::map< std::string, Service::WSHandler > asio_handlers_
Handlers registered through the WebSockAPI::Service object.
A module that provide a websocket interface to Leosac.
std::map< websocketpp::connection_hdl, APIPtr, std::owner_less< websocketpp::connection_hdl > > ConnectionAPIMap
This is the header file for a generated source file, GitSHA1.cpp.
ConnectionAPIMap connection_session_
WebSockAPIModule & module_
A reference to the module.
void finalize_audit(const Audit::IWSAPICallPtr &audit, ServerMessage &msg)
Extract values from the msg and finalizes the audit object with them.
boost::optional< ServerMessage > handle_request(APIPtr api_handle, const ClientMessage &msg, Audit::IAuditEntryPtr)
Handle a request.
std::shared_ptr< DBService > DBServicePtr
bool register_asio_handler(const Service::WSHandler &handler, const std::string &name)
This function block the calling thread until the WebSocket thread has processed the handler registrat...
std::function< boost::optional< json >(const RequestContext &)> WSHandler
bool has_handler(const std::string &name) const
Returns true if an handler named name already exists.
The implementation class that runs the websocket server.
std::shared_ptr< IWSAPICall > IWSAPICallPtr
void on_close(websocketpp::connection_hdl hdl)
CoreUtilsPtr core_utils()
Retrieve the CoreUtils pointer.
DBServicePtr dbsrv_
Database service object.
DBPtr db()
Retrieve database handle.
std::shared_ptr< APISession > APIPtr
ClientMessage parse_request(const json &in)
Create a ClientMessage object from a json request.
void start_shutdown()
Start the process of shutting down the server.
std::unique_ptr< boost::asio::io_service::work > work_
Work used to keep the io_service alive while someone has a reference to (WS) Service object.
This is the application-level object that provide the API.
DBServicePtr dbsrv()
Retrieve database service pointer.
void attempt_unregister_ws_service()
std::shared_ptr< CoreUtils > CoreUtilsPtr
void register_crud_handler(const std::string &resource_name, CRUDResourceHandler::Factory factory)
An internal helper function to register a CRUD resource handler.
std::map< std::string, json(APISession::*)(const json &)> handlers_
This maps (string) command name to API method.