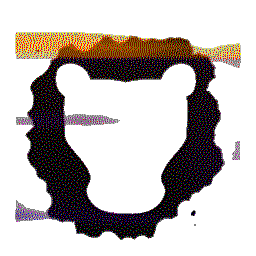 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <boost/date_time/posix_time/posix_time.hpp>
41 #pragma db object optimistic
55 const std::string &
id()
const;
61 const std::string &
token()
const;
86 using namespace std::chrono;
87 auto duration_second = duration_cast<seconds>(duration);
89 auto now = boost::posix_time::second_clock::local_time();
90 expiration_ = now + boost::posix_time::seconds(duration_second.count());
100 #pragma db type("VARCHAR(128)")
107 #pragma db on_delete(cascade)
An authentication token used for authenticating a user against Leosac.
std::shared_ptr< User > UserPtr
bool is_valid() const
Check if the token is still active.
const std::string & id() const
Return the token identifier.
This is the header file for a generated source file, GitSHA1.cpp.
Exception class for LEOSAC Project related errors.
boost::posix_time::ptime expiration() const
Retrieve the unix timestamp at which the token will/has expire(d).
void expire_in(const T &duration)
Set the expiration point of the token to be now + duration.
boost::posix_time::ptime expiration_
UserPtr owner_
The user owning the token.
UserPtr owner() const
Retrieve a shared_ptr to the user owning the token.
std::string token_
The string representation of the token.
const std::string & token() const
Retrieve the string representation of the token.