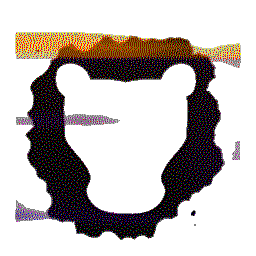 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 std::string
const &gpio_name,
int blink_duration,
29 , frontend_(ctx,
zmqpp::socket_type::rep)
30 , backend_(ctx,
zmqpp::socket_type::req)
31 , gpio_(ctx, gpio_name)
32 , default_blink_duration_(blink_duration)
33 , default_blink_speed_(blink_speed)
34 , stmachine_(std::ref(gpio_))
37 backend_.connect(
"inproc://" + gpio_name);
56 if (frame1 ==
"STATE")
60 if (frame1 ==
"ON" || frame1 ==
"OFF" || frame1 ==
"TOGGLE")
67 else if (frame1 ==
"BLINK")
69 else if (frame1 ==
"FAST_TO_SLOW")
74 {5000, 1000}, {2100, 700}, {1000, 100},
85 DEBUG(
"UPDATING LED");
110 if (msg->parts() > 1)
115 if (msg->parts() > 2)
117 *msg >> event_blink.
speed;
131 st << static_cast<int64_t>(
stmachine_.led_state_.duration)
132 <<
static_cast<int64_t
>(
stmachine_.led_state_.speed);
int64_t default_blink_speed_
std::vector< std::pair< int, int > > pattern
A vector of <duration, speed> that represents our blinking pattern.
LedBuzzerSM stmachine_
Our state machine that handle blinking, blinking in pattern or doing nothing.
int64_t speed
Period for before blinking (ie switch state every N ms)
int64_t default_blink_duration_
This event is fired when we want to start blinking.
std::chrono::system_clock::time_point next_update()
Time point of the next wanted update.
void update()
Update the object.
void send_state()
Write the current state of the LED device (according to specs) to the frontend_ socket.
void handle_message()
Message received on the rep_ socket.
zmqpp::socket & frontend()
Return the frontend_ socket.
zmqpp::message send_to_backend(zmqpp::message &msg)
Send a message to the backend object (used for ON, OFF, TOGGLE).
Namespace where implementation of Led (or buzzer) support is done.
bool start_blink(zmqpp::message *msg)
Start blinking, this stores the blink_end timepoint and send commands for blinking to happen.
zmqpp::socket frontend_
REP socket to receive LED command.
bool isOn()
Query the value of the GPIO and returns true if the LED is ON.
Hardware::FGPIO gpio_
Facade to the GPIO we use with this LED.
zmqpp::socket backend_
REQ socket to the backend GPIO.
Fired when we update the state machine.
LedBuzzerImpl(zmqpp::context &ctx, const std::string &led_name, const std::string &gpio_name, int blink_duration, int blink_speed)
int64_t duration
Requested duration (in ms) of the blink.
Fired when we want to "play" a pattern.