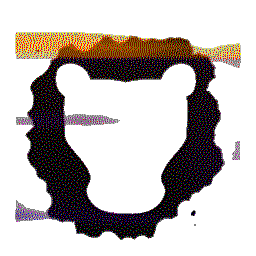 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
22 #include <zmqpp/message.hpp>
27 : gpio_name_(gpio_name)
28 , backend_(ctx,
zmqpp::socket_type::req)
30 backend_.connect(
"inproc://" + gpio_name);
39 msg <<
"ON" << duration.count();
104 assert(rep ==
"OFF");
#define ASSERT_LOG(cond, msg)
bool toggle()
Toggle the GPIO value by sending a message to the backend GPIO impl.
zmqpp::poller poller_
A poller to not wait for infinity in case something went wrong.
const std::string & name() const
Name of the GPIO pin as defined in the configuration file.
bool isOn()
Query the value of the GPIO and returns true if the LED is ON.
bool isOff()
Similar to isOn().
FGPIO(zmqpp::context &ctx, const std::string &gpio_name)
bool turnOff()
Turn the GPIO OFF by sending a message to the backend GPIO impl.
Provides facade classes to hardware device implementation.
zmqpp::socket backend_
A socket to talk to the backend GPIO.
bool turnOn()
Turn the GPIO ON by sending a message to the backend GPIO impl.