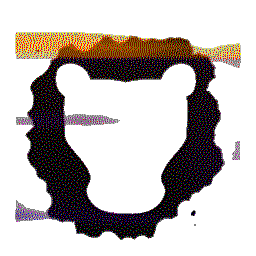 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <zmqpp/poller.hpp>
25 #include <zmqpp/socket.hpp>
86 FLED(zmqpp::context &ctx,
const std::string &led_name);
102 bool turnOn(
int duration);
107 bool turnOn(std::chrono::milliseconds duration);
133 bool blink(std::chrono::milliseconds duration, std::chrono::milliseconds speed);
135 bool blink(
int duration,
int speed);
int64_t speed
Set only if st is BLINKING, it represents the speed of blinking.
enum Leosac::Hardware::FLED::State::@0 st
Internal state of the LED.
bool turnOff()
Turn the LED OFF by sending a message to the backend LED impl.
FLED(zmqpp::context &ctx, const std::string &led_name)
bool isOff()
Similar to isOn().
A Facade to a LED object.
bool isOn()
Query the value of the GPIO and returns true if the LED is ON.
This is the header file for a generated source file, GitSHA1.cpp.
zmqpp::poller poller_
A poller to not wait for infinity in case something went wrong.
zmqpp::socket backend_
A socket to talk to the backend LED.
bool value
Set only if st is BLINKING : value of the LED (true if ON, false otherwise).
State state()
Return the state of the device.
bool toggle()
Toggle the LED value by sending a message to the backend LED impl.
~FLED()=default
Default destructor.
bool isBlinking()
Returns true is the LED is currently blinking.
zmqpp::socket & backend()
Access the backend socket (which is connect to the LED device) to send command directly.
int64_t duration
Set only if st is BLINKING, it represents the total duration of blinking.
bool turnOn()
Turn the LED ON by sending a message to the backend LED impl.
bool blink()
Make the LED blink.