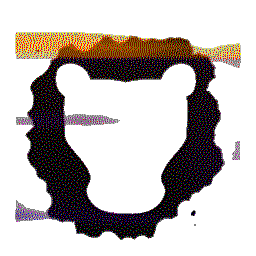 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
25 #include <boost/archive/text_oarchive.hpp>
26 #include <boost/property_tree/ptree_serialization.hpp>
33 boost::property_tree::ptree
const &cfg,
CoreUtilsPtr utils)
39 , control_(ctx,
zmqpp::socket_type::rep)
41 name_ = cfg.get<std::string>(
"name");
62 assert(msg.is_signal());
64 if (sig == zmqpp::signal::stop)
69 "Module receive a message on its pipe that wasn't a signal. Aborting.");
71 throw std::runtime_error(
72 "Module receive a message on its pipe that wasn't a signal. Aborting.");
77 zmqpp::message *out_msg)
const
82 std::ostringstream oss;
83 boost::archive::text_oarchive archive(oss);
84 boost::property_tree::save(archive,
config_, 1);
85 out_msg->add(oss.str());
95 catch (std::exception &e)
97 ERROR(
"Problem while dumping config: " << e.what());
108 if (frame1 ==
"DUMP_CONFIG")
110 zmqpp::message response;
112 assert(msg.remaining() == 1);
115 DEBUG(
"Module " <<
name_ <<
" is dumping config!");
121 ERROR(
"Module received invalid request (" << frame1 <<
"). Aborting.");
123 throw std::runtime_error(
"Invalid request for module.");
133 if (
utils_->config_checker().has_object(obj_name))
136 std::string prefix =
"Configuration Error (module " +
name_ +
") ";
137 ERROR(prefix <<
"Object " << obj_name <<
" cannot be found.");
146 bool res =
utils_->config_checker().has_object(obj_name, type);
151 std::string prefix =
"Configuration Error (module " +
name_ +
") ";
156 ERROR(prefix <<
"GPIO " << obj_name <<
" doesn't exist.");
159 ERROR(prefix <<
"LED " << obj_name <<
" doesn't exist.");
162 ERROR(prefix <<
"BUZZER " << obj_name <<
" doesn't exists.");
165 ERROR(prefix <<
"READER " << obj_name <<
" doesn't exists.");
168 ERROR(prefix <<
"EXTERNAL SERVER " << obj_name <<
" doesn't exists.");
171 ASSERT_LOG(
false, prefix <<
"Missing case in switch: value "
172 <<
static_cast<int>(type) <<
" Need code fix.");
BaseModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
Constructor of BaseModule.
zmqpp::socket & pipe_
A reference to the pair socket that link back to the module manager.
DeviceClass
An enumeration describing the class of the device.
void config_check(const std::string &obj_name, Leosac::Hardware::DeviceClass type)
An helper that checks configuration the existence of some objects.
#define ASSERT_LOG(cond, msg)
ConfigFormat
This enum is used internally, when core request module configuration.
All modules that provides features to Leosac shall be in this namespace.
boost::property_tree::ptree config_
The configuration tree passed to the start_module function.
virtual void handle_pipe()
The base class register the pipe_ socket to its reactor_ so that this function is called when the pip...
zmqpp::reactor reactor_
The reactor object we poll() on in the main loop.
virtual void handle_control()
Handle called when a message on the module's control socket arrives.
CoreUtilsPtr utils_
Pointer to the core utils, which gives access to scheduler and others.
bool is_running_
Boolean indicating whether the main loop should run or not.
virtual void dump_additional_config(zmqpp::message *out) const
Dump additional configuration (for example module specific config file).
virtual void run()
This is the main loop of the module.
std::string format(const std::string &escape_code, const T &in)
Return a string containing the escape code, a string representation of T and the clear escape string.
zmqpp::socket control_
Control REP socket.
std::shared_ptr< CoreUtils > CoreUtilsPtr
void dump_config(ConfigManager::ConfigFormat fmt, zmqpp::message *out_msg) const
Fills a message with the module's configuration information.