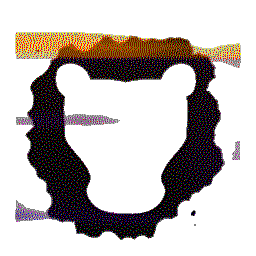 |
Leosac
0.8.0
Open Source Access Control
|
std::shared_ptr< GPIO > GPIOPtr
DeviceClass
An enumeration describing the class of the device.
std::shared_ptr< LED > LEDPtr
This is the header file for a generated source file, GitSHA1.cpp.
std::shared_ptr< ExternalMessage > ExternalMessagePtr
std::shared_ptr< ExternalServer > ExternalServerPtr
std::shared_ptr< Device > DevicePtr
Abstraction of an External Messaging device.
Abstraction of LED device attributes.
Abstraction of an External Server device.
Abstraction of Buzzer device attributes.
std::shared_ptr< Buzzer > BuzzerPtr
Abstraction of a GPIO device attributes.
Thin wrapper around boost::uuids::uuid.