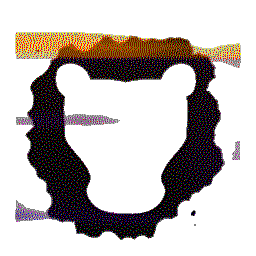 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
27 #include <boost/property_tree/ptree.hpp>
28 #include <zmqpp/zmqpp.hpp>
44 template <
typename UserModule>
51 UserModule module(zmq_ctx, pipe, cfg, utils);
54 pipe->send(zmqpp::signal::ok);
58 catch (
const std::exception &e)
60 ERROR(
"An exception occurred in module code. Leosac will terminate."
62 <<
"Message: " << e.what() << std::endl
116 BaseModule(zmqpp::context &ctx, zmqpp::socket *pipe,
117 const boost::property_tree::ptree &cfg,
CoreUtilsPtr utils);
BaseModule(zmqpp::context &ctx, zmqpp::socket *pipe, const boost::property_tree::ptree &cfg, CoreUtilsPtr utils)
Constructor of BaseModule.
uint64_t gettid()
Return the Linux thread ID.
zmqpp::socket & pipe_
A reference to the pair socket that link back to the module manager.
Base class for module implementation.
DeviceClass
An enumeration describing the class of the device.
void config_check(const std::string &obj_name, Leosac::Hardware::DeviceClass type)
An helper that checks configuration the existence of some objects.
bool start_module_helper(zmqpp::socket *pipe, boost::property_tree::ptree cfg, zmqpp::context &zmq_ctx, CoreUtilsPtr utils)
This function template is a helper wrt writing the start_module function for every module.
ConfigFormat
This enum is used internally, when core request module configuration.
void set_thread_name(const std::string &name)
Set the name of the current thread.
This is the header file for a generated source file, GitSHA1.cpp.
boost::property_tree::ptree config_
The configuration tree passed to the start_module function.
virtual void handle_pipe()
The base class register the pipe_ socket to its reactor_ so that this function is called when the pip...
zmqpp::reactor reactor_
The reactor object we poll() on in the main loop.
virtual void handle_control()
Handle called when a message on the module's control socket arrives.
CoreUtilsPtr utils_
Pointer to the core utils, which gives access to scheduler and others.
bool is_running_
Boolean indicating whether the main loop should run or not.
virtual void dump_additional_config(zmqpp::message *out) const
Dump additional configuration (for example module specific config file).
const char * get_module_name()
virtual ~BaseModule()=default
virtual void run()
This is the main loop of the module.
std::string format(const std::string &escape_code, const T &in)
Return a string containing the escape code, a string representation of T and the clear escape string.
zmqpp::socket control_
Control REP socket.
zmqpp::context & ctx_
A reference to the ZeroMQ context in case you need it to create additional socket.
std::shared_ptr< CoreUtils > CoreUtilsPtr
void dump_config(ConfigManager::ConfigFormat fmt, zmqpp::message *out_msg) const
Fills a message with the module's configuration information.