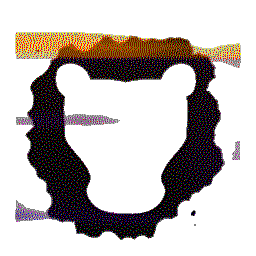 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 #include <boost/algorithm/string/join.hpp>
38 std::string
const &auth_ctx_name,
39 const std::list<std::string> &auth_sources_names,
40 std::string
const &auth_target_name,
41 std::string
const &input_file,
44 , bus_push_(ctx,
zmqpp::socket_type::push)
45 , bus_sub_(ctx,
zmqpp::socket_type::sub)
46 , name_(auth_ctx_name)
47 , target_name_(auth_target_name)
48 , file_path_(input_file)
49 , core_utils_(core_utils)
51 bus_push_.connect(
"inproc://zmq-bus-pull");
52 bus_sub_.connect(
"inproc://zmq-bus-pub");
56 INFO(
"Auth instance (" << auth_ctx_name <<
") subscribe to "
57 << boost::algorithm::join(auth_sources_names,
", "));
58 for (
const auto &auth_source : auth_sources_names)
59 bus_sub_.subscribe(
"S_" + auth_source);
64 INFO(
"AuthFileInstance down");
69 using namespace Colorize;
71 zmqpp::message auth_result_msg;
77 auth_result_msg << (
"S_" +
name_);
87 if (auth_result.success)
113 std::lock_guard<std::mutex> guard(
mutex_);
117 DEBUG(
"Auth source OK... will map");
118 mapper_->mapToUser(auth_source);
119 DEBUG(
"Mapping done");
124 INFO(
"Using Credential: " << cred_serialized);
125 auto profile =
mapper_->buildProfile(auth_source);
129 INFO(
"No profile was created from this auth source message.");
131 return {
false,
nullptr,
nullptr};
137 profile->isAccessGranted(std::chrono::system_clock::now(),
nullptr),
138 profile, auth_source->owner().get_eager()};
143 return {profile->isAccessGranted(std::chrono::system_clock::now(), t),
144 profile, auth_source->owner().get_eager()};
147 catch (std::exception &e)
149 WARN(
"Exception when handling authentication request.");
152 return {
false,
nullptr,
nullptr};
158 std::stringstream buffer;
178 auto self = shared_from_this();
183 auto mapper = std::make_shared<FileAuthSourceMapper>(file_path);
185 std::lock_guard<std::mutex> guard(self->mutex_);
186 self->mapper_ = mapper;
187 INFO(
"AuthFileInstance config reloaded.");
191 catch (
const std::exception &e)
193 WARN(
"Problem when reloading AuthFileInstance configuration: "
203 auto cp = msg.copy();
Holds classes relevant to the Authentication and Authorization subsystem.
Represent an object that we are authorizing against (a door).
void reload_auth_config()
Schedule an asynchronous reload of the module configuration file.
static SecurityContext & instance()
virtual Cred::ICredentialPtr create(zmqpp::message *msg)
Create a Credential object from a message.
const std::string & auth_file_name() const
Return the name of the file associated with the authenticator.
FileAuthSourceMapperPtr mapper_
Authentication config file parser.
std::string target_name_
Name of the target we auth against.
zmqpp::socket bus_push_
Socket to write to the bus.
std::string file_path_
Path to the auth data file.
void log_exception(const std::exception &e, int level=0)
Recursively log exceptions using the logging macro.
static std::string serialize(const Cred::ICredential &in, const SecurityContext &sc)
std::string underline(const T &in)
std::string auth_file_content() const
Return the content of the configuration file use for user/group and permission mapping.
std::shared_ptr< AuthTarget > AuthTargetPtr
bool handle_kernel_message(const zmqpp::message &msg)
Handle the message if its from Leosac's kernel, or does nothing.
std::string bold(const T &in)
This class is some kind of factory to create IAuthenticationSource object from a zmqpp::message sent ...
std::mutex mutex_
A mutex used only internally.
AuthFileInstance(zmqpp::context &ctx, const std::string &auth_ctx_name, const std::list< std::string > &auth_sources_names, const std::string &auth_target_name, const std::string &input_file, CoreUtilsPtr core_utils)
Create a new Authenticator that watch a device and emit authentication message.
std::string green(const T &in)
static TaskPtr build(const Callable &callable)
zmqpp::socket & bus_sub()
Returns the socket subscribed to the message bus.
void handle_bus_msg()
Something happened on the bus that we have interest into.
AuthResult handle_auth(zmqpp::message *msg) noexcept
Prepare auth source object, map them to profile and check if access is granted.
std::string red(const T &in)
std::shared_ptr< ICredential > ICredentialPtr
std::string name_
Name of this auth context instance.
Authentication backend modules live here.
std::shared_ptr< CoreUtils > CoreUtilsPtr
zmqpp::socket bus_sub_
Socket to read from the bus.
Use a file to map auth source (card, PIN, etc) to user.