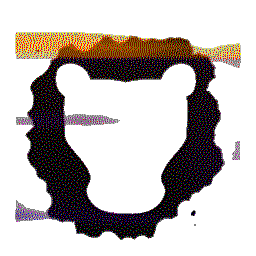 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
41 explicit AuthTarget(
const std::string target_name);
43 const std::string &
name()
const;
44 void name(
const std::string &new_name);
52 bool is_always_open(
const std::chrono::system_clock::time_point &tp)
const;
57 bool is_always_closed(
const std::chrono::system_clock::time_point &tp)
const;
76 void gpio(std::unique_ptr<Hardware::FGPIO> new_gpio);
80 void exitreq_gpio(std::unique_ptr<Hardware::FGPIO> new_gpio);
88 void contact_gpio(std::unique_ptr<Hardware::FGPIO> new_gpio);
103 std::unique_ptr<Hardware::FGPIO>
gpio_;
void add_always_open_sched(const Tools::IScheduleCPtr &sched)
Represent an object that we are authorizing against (a door).
Hardware::FGPIO * exitreq_gpio()
std::vector< Tools::IScheduleCPtr > always_close_
std::chrono::milliseconds contact_duration()
const std::string & name() const
Hardware::FGPIO * gpio()
Returns the pointer to the optional FGPIO associated with the door.
A Facade to a GPIO object.
std::shared_ptr< AuthTarget > AuthTargetPtr
This is the header file for a generated source file, GitSHA1.cpp.
std::unique_ptr< Hardware::FGPIO > gpio_
Optional GPIO associated with the door.
virtual ~AuthTarget()=default
Hardware::FGPIO * contact_gpio()
void add_always_close_sched(const Tools::IScheduleCPtr &sched)
std::unique_ptr< Hardware::FGPIO > exitreq_gpio_
Optional Exit Req GPIO associated with the door.
std::chrono::milliseconds exitreq_duration()
std::vector< Tools::IScheduleCPtr > always_open_
void resetToExpectedState(const std::chrono::system_clock::time_point &tp)
bool is_always_closed(const std::chrono::system_clock::time_point &tp) const
Check whether the door is in "always closed" mode at the given time point.
std::chrono::milliseconds exitreq_duration_
Duration for the Exit Req to keep the door open.
std::chrono::milliseconds contact_duration_
Duration for the Contact Door Sensor to be ignored before triggering an alarm.
AuthTarget(const std::string target_name)
std::unique_ptr< Hardware::FGPIO > contact_gpio_
Optional Contact Door Sensor GPIO associated with the door.
bool is_always_open(const std::chrono::system_clock::time_point &tp) const
Check whether the door is in "always open" mode at the given time point.