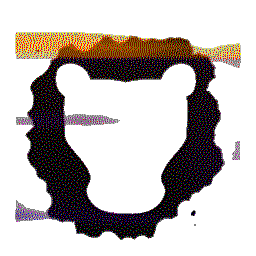 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 #include <boost/property_tree/ptree.hpp>
35 #include <unordered_map>
76 std::vector<Leosac::Auth::GroupPtr>
groups()
const override;
108 void load_users(
const boost::property_tree::ptree &users);
114 void load_schedules(
const boost::property_tree::ptree &schedules);
120 void map_schedules(
const boost::property_tree::ptree &schedules_mapping);
125 void load_groups(
const boost::property_tree::ptree &group_mapping);
149 merge_profiles(
const std::vector<Leosac::Auth::IAccessProfilePtr> profiles);
162 std::map<std::string, Leosac::Auth::UserPtr>
users_;
167 std::map<std::string, Leosac::Auth::GroupPtr>
groups_;
172 std::unordered_map<std::string, Leosac::Cred::RFIDCardPtr>
rfid_cards_;
178 std::unordered_map<std::string, Leosac::Cred::PinCodePtr>
pin_codes_;
190 std::unordered_map<std::string, Leosac::Cred::ICredentialPtr>
id_to_cred_;
204 std::vector<Leosac::Auth::DoorPtr>
doors_;
std::map< std::string, Leosac::Auth::GroupPtr > groups_
Maps group name to object.
void add_cred_to_id_map(Leosac::Cred::ICredentialPtr credential)
Store the credential to the id <-> credential map if the id is non-empty.
Cred::ICredentialPtr find_cred_by_alias(const std::string &alias)
Lookup a credentials by ID.
Credentials composed of an RFIDCard and a PIN code.
std::shared_ptr< FileAuthSourceMapper > FileAuthSourceMapperPtr
std::shared_ptr< User > UserPtr
std::unordered_map< std::string, Leosac::Cred::PinCodePtr > pin_codes_
Maps PIN code to object.
std::shared_ptr< IAccessProfile > IAccessProfilePtr
void load_credentials(const boost::property_tree::ptree &credentials)
Eager loading of credentials to avoid walking through the ptree whenever we have to grant/deny an acc...
Leosac::Auth::SimpleAccessProfilePtr build_group_profile(Leosac::Auth::GroupPtr g)
Tools::XmlNodeNameEnforcer xmlnne_
std::map< std::pair< std::string, std::string >, Leosac::Cred::RFIDCardPinPtr > rfid_cards_pin
Maps WiegandCard + PIN code to object.
virtual Leosac::Auth::IAccessProfilePtr buildProfile(Leosac::Cred::ICredentialPtr cred)
Build an AccessProfile object given a Credential.
void load_groups(const boost::property_tree::ptree &group_mapping)
Extract group membership.
void load_schedules(const boost::property_tree::ptree &schedules)
Load the schedules information from the config tree.
This is the header file for a generated source file, GitSHA1.cpp.
virtual void mapToUser(Leosac::Cred::ICredentialPtr auth_source)
Must map the ICredential data to a User.
std::shared_ptr< Group > GroupPtr
std::shared_ptr< SimpleAccessProfile > SimpleAccessProfilePtr
std::vector< Leosac::Auth::GroupPtr > groups() const override
Return the groups this mapper is aware of.
std::unordered_map< std::string, Leosac::Cred::RFIDCardPtr > rfid_cards_
Maps card_id to object.
std::map< std::string, Leosac::Auth::UserPtr > users_
Maps user id (or name) to object.
std::string config_file_
Store the name of the configuration file.
Base class to perform abstracted mapping operation over various AuthSource object.
virtual void visit(::Leosac::Cred::RFIDCard &src) override
Try to map a wiegand card_id to a user.
std::unordered_map< std::string, Leosac::Cred::ICredentialPtr > id_to_cred_
Maps credentials ID (from XML) to object.
Tools::XmlScheduleLoader xml_schedules_
FileAuthSourceMapper(const std::string &auth_file)
Leosac::Auth::SimpleAccessProfilePtr build_cred_profile(Leosac::Cred::ICredentialPtr c)
std::shared_ptr< ICredential > ICredentialPtr
Leosac::Auth::ValidityInfo extract_credentials_validity(const boost::property_tree::ptree &node)
std::shared_ptr< RFIDCardPin > RFIDCardPinPtr
void map_schedules(const boost::property_tree::ptree &schedules_mapping)
Interpret the schedule mapping content of the config file.
Leosac::Auth::IAccessProfilePtr merge_profiles(const std::vector< Leosac::Auth::IAccessProfilePtr > profiles)
Merge a bunch of profiles together and returns a new profile.
void load_users(const boost::property_tree::ptree &users)
Load users from configuration tree, storing them in the users_ map.
std::vector< Tools::ScheduleMappingPtr > mappings_
List of mappings defined in the configuration file.
std::vector< Leosac::Auth::GroupPtr > get_user_groups(Leosac::Auth::UserPtr u)
Naive method that bruteforce groups to try to find membership for an user.
Use a file to map auth source (card, PIN, etc) to user.
Leosac::Auth::SimpleAccessProfilePtr build_user_profile(Leosac::Auth::UserPtr u)
Build an access for a user.
A simple class that stores (and can be queried for) the validity of some objects.
std::vector< Leosac::Auth::DoorPtr > doors_
We store doors object, but really we only use the name property.