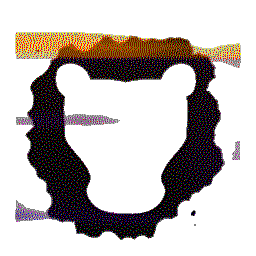 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include "core/audit/AuditEntry_odb.h"
30 #include "tools/LogEntry_odb.h"
34 #include <odb/pgsql/query.hxx>
47 return std::make_unique<AuditGet>(ctx);
56 using namespace Tools;
57 using namespace JSONUtil;
58 using Query = odb::query<Audit::AuditEntry>;
66 odb::transaction t(db->begin());
69 rep[
"meta"][
"count"] = view.
count;
72 rep[
"meta"][
"total_page"] =
73 (view.
count / page_size) + (view.
count % page_size ? 1 : 0);
76 rep[
"meta"][
"total_page"] = 0;
80 rep[
"data"] = json::array();
81 for (
const auto &audit : ret)
85 rep[
"data"].push_back(audit_json);
97 std::vector<ActionActionParam> perm_;
107 std::stringstream request_builder;
110 request_builder <<
" ORDER BY id DESC";
111 request_builder <<
" LIMIT " << page_size;
112 request_builder <<
" OFFSET " << page_size * (page - 1);
113 DEBUG(
"QUERY: " << request_builder.str());
114 return request_builder.str();
119 for (
const auto &c : str)
121 if (c !=
':' && !isalpha(c))
129 std::stringstream request_builder;
131 if (req.find(
"enabled_type") != req.end() && req.at(
"enabled_type").is_array() &&
132 req.at(
"enabled_type").size())
134 const auto &enabled_types = req.at(
"enabled_type");
135 request_builder <<
"WHERE typeid IN (";
136 for (
size_t i = 0; i < enabled_types.size(); ++i)
138 auto enabled_type = enabled_types[i].get<std::string>();
142 BUILD_STR(
"Audit type string is invalid: " << enabled_type));
144 request_builder <<
"'" << enabled_type <<
"'";
145 if (i != enabled_types.size() - 1)
146 request_builder <<
",";
148 request_builder <<
")";
152 request_builder <<
"1 = 1";
154 return request_builder.str();
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
#define BUILD_STR(param)
Internal macro.
std::string build_in_clause(const json &req) const
Build the "WHERE typeid IN (...)" string based on the enabled types.
std::vector< ActionActionParam > required_permission(const json &req) const override
Return a list of "Action" / "ActionParam" that must pass before the request is processed.
std::string build_request_string(const json &req, int page, int page_size) const
bool is_stringtype_sane(const std::string &str) const
Check that a given string representing an audit type is sane.
std::shared_ptr< odb::database > DBPtr
All modules that provides features to Leosac shall be in this namespace.
odb::query< Tools::LogEntry > Query
The base class for API method handler implementation.
static MethodHandlerUPtr create(RequestContext)
This is the header file for a generated source file, GitSHA1.cpp.
A base class for Leosac specific exception.
AuditGet(RequestContext ctx)
std::unique_ptr< MethodHandler > MethodHandlerUPtr
UserSecurityContext & security_context()
#define LEOSAC_ENFORCE_ARGUMENT(cond, var, msg)
A macro to perform argument checking that results in an exception being thrown on failure.
static json serialize(const Audit::IAuditEntry &in, const SecurityContext &sc)
Perform deep serialization of the AuditEntry in.
@ AUDIT_READ
Read the audit log.
Holds valuable pointer to provide context to a request.
Implementation of IAuditEntry, backed by ODB.
virtual json process_impl(const json &req) override
The API method implementation.