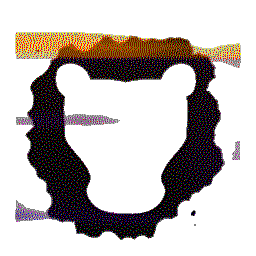 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
57 "The serializer didn't set a type.");
63 : security_context_(sc)
121 const auto &audit_entry = assert_cast<const Audit::IAuditEntry &>(visitable);
125 "Cannot retrieve Audit::Serializer::JSONService. Since this a "
126 "core service, something must be very wrong.");
128 result_ = service->serialize(audit_entry, security_context_);
static SecurityContext & instance()
static json serialize(const Audit::IGroupEvent &in, const SecurityContext &sc)
Interface that describes an Audit object for group related event.
Interface that describes an Audit object for door related event.
static json serialize(const Audit::IUpdateEvent &in, const SecurityContext &sc)
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
Audit interface to Credential related events.
#define ASSERT_LOG(cond, msg)
static json serialize(const Audit::ICredentialEvent &in, const SecurityContext &sc)
static json serialize(const Audit::IZoneEvent &in, const SecurityContext &sc)
json result_
Store the result here because we can't return from the visit() method.
Interface that describes an Audit object when a user-related event happens.
static json serialize(const Audit::IUserEvent &in, const SecurityContext &sc)
This is the header file for a generated source file, GitSHA1.cpp.
static json serialize(const Audit::IWSAPICall &in, const SecurityContext &sc)
Non static helper that can visit a core audit object.
virtual void visit(const Audit::IUserEvent &t) override
HelperSerialize(const SecurityContext &sc)
Audit interface to Schedule related events.
static std::string type_name(const Audit::IAuditEntry &in)
Returns the "type-name" of the audit entry.
Base interface to Audit object.
static json serialize(const Audit::IUserGroupMembershipEvent &in, const SecurityContext &sc)
virtual void cannot_visit(const Tools::IVisitable &visitable) override
Called when no "hardcoded" audit type match, this method will delegate to runtime-registered serializ...
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
Interface that describes an Audit object for zone related event.
Interface for update-related event.
static json serialize(const Audit::IAuditEntry &in, const SecurityContext &sc)
Perform deep serialization of the AuditEntry in.
Interface to audit object that take care of tracking user/group membership change.
static json serialize(const Audit::IScheduleEvent &in, const SecurityContext &sc)
A SecurityContext is used to query permission while doing an operation.
This service manages runtime registered serializer that target AuditEntry object.
An audit entry dedicated to tracing API call.
static json serialize(const Audit::IDoorEvent &in, const SecurityContext &sc)