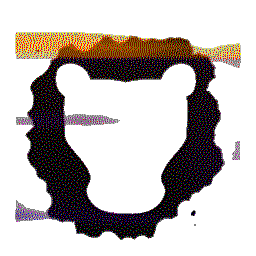 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
43 <<
" but we expected 26)");
51 for (
int i = 9; i < 26; ++i)
53 unsigned int v = ((
reader_->
buffer()[i / 8] >> (7 - i % 8)) & 0x01);
54 n |= v << (15 - (i - 9));
59 WARN(
"Invalid Pin Code");
62 pin_ = std::to_string(n);
74 assert(
pin_.length());
An implementation class that represents a Wiegand Reader.
@ WIEGAND_PIN
Message formatting when using a simple PIN code.
virtual const std::string & get_pin() const override
Retrieve the pin code that was read from the reader.
Interface for a strategy that read a PIN code.
virtual bool completed() const override
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...
void read_reset()
Reset the "read state" of the reader, effectively cleaning the wiegand-bit-buffer and resetting the c...
int counter() const
Returns the number of bits read.
const unsigned char * buffer() const
Return a pointer to internal buffer memory.
WiegandPinBuffered(WiegandReaderImpl *reader)
Create a strategy that read 4bits-per-key PIN code.
virtual void signal(zmqpp::socket &sock) override
Tells the strategy implementation to send a message to the application containing the received creden...
virtual void reset() override
Reset the strategy, meaning that the next time timeout() is called the behavior should be the same th...
virtual void timeout() override
This is called when the module detect a timeout.
Provide support for Wiegand devices.
WiegandReaderImpl * reader_
const std::string & name() const
Returns the name of this reader.