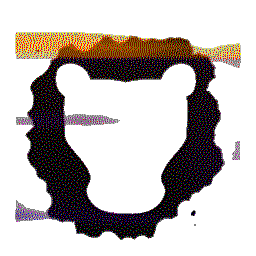 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include "zmqpp/zmqpp.hpp"
24 #include <boost/any.hpp>
34 class WiegandReaderImpl;
37 class WiegandStrategy;
85 virtual void signal(zmqpp::socket &sock) = 0;
94 virtual void reset() = 0;
An implementation class that represents a Wiegand Reader.
std::unique_ptr< WiegandStrategy > WiegandStrategyUPtr
virtual void timeout()=0
This is called when the module detect a timeout.
virtual void set_reader(WiegandReaderImpl *new_ptr)
Update the pointer that points back to the associated reader.
This is the header file for a generated source file, GitSHA1.cpp.
virtual ~WiegandStrategy()=default
The multiple modes available to wiegand reader are implemented through the strategy pattern.
WiegandStrategy(WiegandReaderImpl *reader)
WiegandReaderImpl * reader_
virtual void signal(zmqpp::socket &sock)=0
Tells the strategy implementation to send a message to the application containing the received creden...
virtual void reset()=0
Reset the strategy, meaning that the next time timeout() is called the behavior should be the same th...
virtual bool completed() const =0
Did the strategy gather needed data? If this function returns true, that means that the strategy impl...