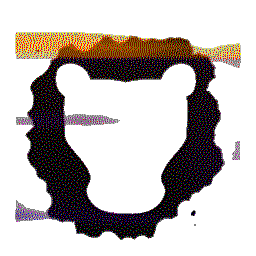 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
32 const std::string &reader_name,
33 const std::string &data_high_pin,
34 const std::string &data_low_pin,
35 const std::string &green_led_name,
36 const std::string &buzzer_name,
37 std::unique_ptr<WiegandStrategy> strategy)
38 : bus_sub_(ctx,
zmqpp::socket_type::sub)
39 , sock_(ctx,
zmqpp::socket_type::rep)
40 , bus_push_(ctx,
zmqpp::socket_type::push)
45 , strategy_(std::move(strategy))
47 bus_sub_.connect(
"inproc://zmq-bus-pub");
48 bus_push_.connect(
"inproc://zmq-bus-pull");
60 if (!green_led_name.empty())
61 green_led_ = std::make_unique<FLED>(ctx, green_led_name);
63 if (!buzzer_name.empty())
64 buzzer_ = std::make_unique<FBuzzer>(ctx, buzzer_name);
72 : bus_sub_(std::move(o.bus_sub_))
73 , sock_(std::move(o.sock_))
74 , bus_push_(std::move(o.bus_push_))
75 , name_(std::move(o.name_))
76 , strategy_(std::move(o.strategy_))
112 WARN(
"Received too many interrupt. Resetting current counter.");
138 assert(str ==
"GREEN_LED" || str ==
"BEEP" || str ==
"BEEP_ON" ||
140 if (str ==
"GREEN_LED")
151 assert(str ==
"OK" || str ==
"KO");
152 sock_.send(str ==
"OK" ?
"OK" :
"KO");
154 else if (str ==
"BEEP")
156 assert(msg.parts() == 2);
164 bool ret =
buzzer_->turnOn(std::chrono::milliseconds(duration));
165 ASSERT_LOG(ret,
"Turning the buzzer ON failed.");
168 else if (str ==
"BEEP_ON")
176 ASSERT_LOG(ret,
"Turning the buzzer ON failed.");
179 else if (str ==
"BEEP_OFF")
187 ASSERT_LOG(ret,
"Turning the buzzer OFF failed.");
void handle_request()
Someone sent a request.
Holds classes relevant to the Authentication and Authorization subsystem.
std::unique_ptr< Hardware::FBuzzer > buzzer_
Facade to the buzzer object.
int counter_
Count the number of bits received from GPIOs.
std::string topic_low_
ZMQ topic-string to interrupt on LOW gpio (low gpio's name)
An implementation class that represents a Wiegand Reader.
#define ASSERT_LOG(cond, msg)
void handle_bus_msg()
Something happened on the bus.
void timeout()
Timeout (no more data burst to handle).
std::unique_ptr< Strategy::WiegandStrategy > strategy_
Concrete implementation of the reader mode.
zmqpp::socket sock_
REP socket to receive command on.
zmqpp::socket bus_push_
Socket to write to the message bus.
void read_reset()
Reset the "read state" of the reader, effectively cleaning the wiegand-bit-buffer and resetting the c...
int counter() const
Returns the number of bits read.
const unsigned char * buffer() const
Return a pointer to internal buffer memory.
Provide support for Wiegand devices.
std::string topic_high_
ZMQ topic-string for interrupt on HIGH gpio (high gpio's name)
std::array< uint8_t, 16 > buffer_
Buffer to store incoming bits from high and low gpios.
WiegandReaderImpl(zmqpp::context &ctx, const std::string &reader_name, const std::string &data_high_pin, const std::string &data_low_pin, const std::string &green_led_name, const std::string &buzzer_name, std::unique_ptr< Strategy::WiegandStrategy > strategy)
Create a new implementation of a Wiegand Reader.
Provides facade classes to hardware device implementation.
zmqpp::socket bus_sub_
Socket that allows the reader to listen to the application BUS.
const std::string & name() const
Returns the name of this reader.
std::string name_
Name of the device (defined in configuration)
std::unique_ptr< Hardware::FLED > green_led_
Facade to control the reader green led.