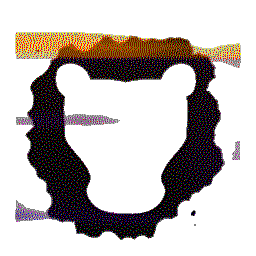 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include "core/auth/User_odb.h"
27 #include "tools/Schedule_odb.h"
29 #include <date/date.h>
37 std::set<Tools::ScheduleId> schedule_ids;
41 auto loaded = mapping.load();
42 ASSERT_LOG(loaded,
"Cannot load. Need to investigate.");
43 schedule_ids.insert(loaded->schedule_id());
45 for (
const auto &
id : schedule_ids)
47 json sched_info = {{
"id",
id}, {
"type",
"schedule"}};
48 schedules.push_back(sched_info);
53 {
"type",
"credential"},
56 {
"alias", in.
alias()},
58 {
"validity-enabled", in.
validity().is_enabled()},
64 serialized[
"relationships"][
"owner"] = {
65 {
"data", {{
"id", in.
owner_id()}, {
"type",
"user"}}}};
67 serialized[
"relationships"][
"schedules"] = {{
"data", schedules}};
74 using namespace JSONUtil;
95 out.
owner(std::shared_ptr<Auth::User>());
std::chrono::system_clock::time_point extract_with_default(const nlohmann::json &obj, const std::string &key, const std::chrono::system_clock::time_point &tp)
Extract an ISO 8601 datetime string from a json object.
ServiceRegistry & get_service_registry()
A function to retrieve the ServiceRegistry from pretty much anywhere.
virtual std::vector< Tools::ScheduleMappingLWPtr > lazy_schedules_mapping() const =0
Retrieve the lazy_weak_ptr to ScheduleMapping that map this credential.
virtual Auth::UserId owner_id() const =0
Returns the id of the owner, or 0 if there is no owner (or the owner has no id).
virtual void validity(const Auth::ValidityInfo &)=0
Provide the validity info object to the credential.
#define ASSERT_LOG(cond, msg)
std::shared_ptr< odb::database > DBPtr
virtual std::string description() const =0
An optional description / notes for the credential.
Provides various database-related services to consumer.
virtual Auth::UserLPtr owner() const =0
Retrieve the owner of the credential.
This is the header file for a generated source file, GitSHA1.cpp.
odb::lazy_shared_ptr< User > UserLPtr
static json serialize(const Cred::ICredential &in, const SecurityContext &sc)
Base interface for credential objects.
static void unserialize(Cred::ICredential &out, const json &in, const SecurityContext &sc)
Auth::ValidityInfo extract_validity_with_default(const nlohmann::json &obj, const std::string &base_key, const Auth::ValidityInfo &def)
Extract fields representing a ValidityInfo object.
std::shared_ptr< ServiceInterface > get_service() const
Retrieve the service instance implementing the ServiceInterface, or nullptr if no such service was re...
std::string format(const std::string &escape_code, const T &in)
Return a string containing the escape code, a string representation of T and the clear escape string.
virtual std::string alias() const =0
An alias for the credential.
virtual CredentialId id() const =0
Retrieve the identifier of the credential.
virtual size_t odb_version() const =0
Credentials are "optimistic" object (wrt ODB).
A SecurityContext is used to query permission while doing an operation.
A simple class that stores (and can be queried for) the validity of some objects.